@ -1,15 +0,0 @@ | |||
# Top-most editorconfig file | |||
root = true | |||
[*] | |||
end_of_line = lf | |||
tab_width = 2 | |||
indent_size = 2 | |||
indent_style = space | |||
[Makefile] | |||
tab_width = 8 | |||
indent_size = 8 | |||
indent_style = tab | |||
@ -0,0 +1,98 @@ | |||
# -*- mode: sh; sh-indentation: 4; indent-tabs-mode: nil; sh-basic-offset: 4; -*- | |||
# Almost all code borrowed from Zshell's _make function | |||
# | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
local -a TARGETS | |||
.make-expandVars() { | |||
local open close var val front='' rest=$1 | |||
while [[ $rest == (#b)[^$]#($)* ]]; do | |||
front=$front${rest[1,$mbegin[1]-1]} | |||
rest=${rest[$mbegin[1],-1]} | |||
case $rest[2] in | |||
($) # '$$'. may not appear in target and variable's value | |||
front=$front\$\$ | |||
rest=${rest[3,-1]} | |||
continue | |||
;; | |||
(\() # Variable of the form $(foobar) | |||
open='(' | |||
close=')' | |||
;; | |||
({) # ${foobar} | |||
open='{' | |||
close='}' | |||
;; | |||
([[:alpha:]]) # $foobar. This is exactly $(f)oobar. | |||
open='' | |||
close='' | |||
var=$rest[2] | |||
;; | |||
(*) # bad parameter name | |||
print -- $front$rest | |||
return 1 | |||
;; | |||
esac | |||
if [[ -n $open ]]; then | |||
if [[ $rest == \$$open(#b)([[:alnum:]_]##)(#B)$close* ]]; then | |||
var=$match | |||
else # unmatched () or {}, or bad parameter name | |||
print -- $front$rest | |||
return 1 | |||
fi | |||
fi | |||
val='' | |||
if [[ -n ${VAR_ARGS[(i)$var]} ]]; then | |||
val=${VAR_ARGS[$var]} | |||
else | |||
if [[ -n $opt_args[(I)(-e|--environment-overrides)] ]]; then | |||
if [[ $parameters[$var] == scalar-export* ]]; then | |||
val=${(P)var} | |||
elif [[ -n ${VARIABLES[(i)$var]} ]]; then | |||
val=${VARIABLES[$var]} | |||
fi | |||
else | |||
if [[ -n ${VARIABLES[(i)$var]} ]]; then | |||
val=${VARIABLES[$var]} | |||
elif [[ $parameters[$var] == scalar-export* ]]; then | |||
val=${(P)var} | |||
fi | |||
fi | |||
fi | |||
rest=${rest//\$$open$var$close/$val} | |||
done | |||
print -- ${front}${rest} | |||
} | |||
.make-parseMakefile () { | |||
local input var val target dep TAB=$'\t' tmp IFS= | |||
while read input | |||
do | |||
case "$input " in | |||
# TARGET: dependencies | |||
# TARGET1 TARGET2 TARGET3: dependencies | |||
([[*?[:alnum:]$][^$TAB:=%]#:[^=]*) | |||
target=$(.make-expandVars ${input%%:*}) | |||
TARGETS+=( ${(z)target} ) | |||
;; | |||
esac | |||
done | |||
} | |||
if [[ -z "${FAST_HIGHLIGHT[chroma-make-cache]}" || $(( EPOCHSECONDS - FAST_HIGHLIGHT[chroma-make-cache-born-at] )) -gt 7 ]]; then | |||
.make-parseMakefile | |||
FAST_HIGHLIGHT[chroma-make-cache-born-at]="$EPOCHSECONDS" | |||
FAST_HIGHLIGHT[chroma-make-cache]="${(j:;:)TARGETS}" | |||
fi | |||
reply2=( "${(s:;:)FAST_HIGHLIGHT[chroma-make-cache]}" ) | |||
# vim:ft=zsh:et |
@ -0,0 +1,30 @@ | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
# | |||
# $1 - path to the ini file to parse | |||
# $2 - name of output hash (INI by default) | |||
# $3 - prefix for keys in the hash (can be empty) | |||
# | |||
# Writes to given hash under keys built in following way: ${3}<section>_field. | |||
# Values are values from ini file. | |||
local __ini_file="$1" __out_hash="${2:-INI}" __key_prefix="$3" | |||
local IFS='' __line __cur_section="void" __access_string | |||
local -a match mbegin mend | |||
[[ ! -r "$__ini_file" ]] && { builtin print -r "fast-syntax-highlighting: an ini file is unreadable ($__ini_file)"; return 1; } | |||
while read -r -t 1 __line; do | |||
if [[ "$__line" = [[:blank:]]#\;* ]]; then | |||
continue | |||
elif [[ "$__line" = (#b)[[:blank:]]#\[([^\]]##)\][[:blank:]]# ]]; then | |||
__cur_section="${match[1]}" | |||
elif [[ "$__line" = (#b)[[:blank:]]#([^[:blank:]=]##)[[:blank:]]#[=][[:blank:]]#(*) ]]; then | |||
match[2]="${match[2]%"${match[2]##*[! $'\t']}"}" # remove trailing whitespace | |||
__access_string="${__out_hash}[${__key_prefix}<$__cur_section>_${match[1]}]" | |||
: "${(P)__access_string::=${match[2]}}" | |||
fi | |||
done < "$__ini_file" | |||
return 0 | |||
# vim:ft=zsh:sw=4:sts=4:et |
@ -0,0 +1,37 @@ | |||
# -*- mode: sh; sh-indentation: 4; indent-tabs-mode: nil; sh-basic-offset: 4; -*- | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
# | |||
# FAST_HIGHLIGHT hash serves as container for variables that | |||
# prevents creating them in global scope. (P) flag is not used, | |||
# output array is fixed (__lines_list). | |||
# | |||
# $1 - the command, e.g. "git remote"; 2>/dev/null redirection is | |||
# added automatically | |||
# $2 - FAST_HIGHLIGHT field name, e.g. "chroma-git-branches"; two | |||
# additional fields will be used, $2-cache, $2-cache-born-at | |||
# $3 - what to remove from beginning of the lines returned by the | |||
# command | |||
# $4 - cache validity time, default 5 (seconds) | |||
# | |||
# Output: array __lines_list, with output of the command ran | |||
# User should not forget to define this array, the below code | |||
# will only ensure that it's array (can also define a global) | |||
typeset -ga __lines_list | |||
local -a __response | |||
if [[ -z "${FAST_HIGHLIGHT[$2-cache]}" || $(( EPOCHSECONDS - FAST_HIGHLIGHT[$2-cache-born-at] )) -gt ${4:-5} ]]; then | |||
FAST_HIGHLIGHT[$2-cache-born-at]="$EPOCHSECONDS" | |||
__response=( ${${(f)"$(command ${(Qz)1#+} 2>/dev/null)"}#${~3}} ) | |||
[[ "$1" = "+"* ]] && __lines_list+=( "${__response[@]}" ) || __lines_list=( "${__response[@]}" ) | |||
FAST_HIGHLIGHT[$2-cache]="${(j:;:)__response}" | |||
else | |||
# Quoted (s:;:) flag without @ will skip empty elements. It | |||
# still produces array output, interesingly. All this is for | |||
# the trailing ";" above, to skip last, empty element. | |||
[[ "$1" = "+"* ]] && \ | |||
__lines_list+=( "${(@s:;:)FAST_HIGHLIGHT[$2-cache]}" ) || \ | |||
__lines_list=( "${(@s:;:)FAST_HIGHLIGHT[$2-cache]}" ) | |||
fi | |||
# vim:ft=zsh:et:sw=4 |
@ -0,0 +1,60 @@ | |||
# -*- mode: sh; sh-indentation: 4; indent-tabs-mode: nil; sh-basic-offset: 4; -*- | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
# | |||
# It runs given command, which in general will be a git command, | |||
# automatically looking at cache first (a field named *-cache, | |||
# in FAST_HIGHLIGHT), which is valid for 5 seconds, and in case | |||
# of outdated or not existing cache, runs the command, splitting | |||
# on new-lines, first checking if PWD is inside git repository. | |||
# | |||
# FAST_HIGHLIGHT hash serves as container for variables that | |||
# prevents creating them in global scope. (P) flag is not used, | |||
# output array is fixed (__lines_list). | |||
# | |||
# $1 - the command, e.g. "git remote"; 2>/dev/null redirection is | |||
# added automatically | |||
# $2 - FAST_HIGHLIGHT field name, e.g. "chroma-git-branches"; two | |||
# additional fields will be used, $2-cache, $2-cache-born-at | |||
# $3 - what to remove from beginning of the lines returned by the | |||
# command | |||
# $4 - cache validity time, default 5 (seconds) | |||
# | |||
# Output: array __lines_list, with output of the (git) command ran | |||
# User should not forget to define this array, the below code | |||
# will only ensure that it's array (can also define a global) | |||
typeset -ga __lines_list | |||
local -a __response | |||
if [[ $1 == --status ]] { | |||
integer __status=1 | |||
shift | |||
} | |||
if [[ -z ${FAST_HIGHLIGHT[$2-cache]} || $(( EPOCHSECONDS - FAST_HIGHLIGHT[$2-cache-born-at] )) -gt ${4:-5} ]]; then | |||
FAST_HIGHLIGHT[$2-cache-born-at]=$EPOCHSECONDS | |||
if [[ "$(command git rev-parse --is-inside-work-tree 2>/dev/null)" = true ]]; then | |||
__response=( ${${(f)"$(command ${(Qz)${1#+}} 2>/dev/null)"}#$3} ) | |||
integer retval=$? | |||
if (( __status )) { | |||
__response=( $retval ) | |||
__lines_list=( $retval ) | |||
} else { | |||
[[ "$1" = "+"* ]] && \ | |||
__lines_list+=( "${__response[@]}" ) || \ | |||
__lines_list=( "${__response[@]}" ) | |||
} | |||
else | |||
__lines_list=() | |||
fi | |||
FAST_HIGHLIGHT[$2-cache]="${(j:;:)__response}" | |||
else | |||
# Quoted (s:;:) flag without @ will skip empty elements. It | |||
# still produces array output, interesingly. All this is for | |||
# the trailing ";" above, to skip last, empty element. | |||
[[ "$1" = "+"* ]] && \ | |||
__lines_list+=( "${(@s:;:)FAST_HIGHLIGHT[$2-cache]}" ) || \ | |||
__lines_list=( "${(@s:;:)FAST_HIGHLIGHT[$2-cache]}" ) | |||
fi | |||
# vim:ft=zsh:et:sw=4 |
@ -0,0 +1,17 @@ | |||
# $1 - file-descriptor to be read from | |||
# $2 - name of output variable (default: REPLY) | |||
local __in_fd=${1:-0} __out_var=${2:-REPLY} | |||
local -a __tmp | |||
integer __ret=1 __repeat=10 __tmp_size=0 | |||
while sysread -s 65535 -i "$__in_fd" '__tmp[__tmp_size + 1]'; do | |||
(( ( __ret=$? ) == 0 )) && (( ++ __tmp_size )) | |||
(( __ret == 5 )) && { __ret=0; (( --__repeat == 0 )) && break; } | |||
done | |||
: ${(P)__out_var::="${(j::)__tmp}"} | |||
return __ret | |||
# vim: ft=zsh:et:sw=4:sts=4 |
@ -1 +0,0 @@ | |||
.revision-hash export-subst |
@ -0,0 +1,5 @@ | |||
# These are supported funding model platforms | |||
github: psprint | |||
patreon: psprint | |||
ko_fi: psprint |
@ -1,74 +0,0 @@ | |||
--- | |||
name: Tests | |||
on: | |||
push: | |||
paths-ignore: | |||
- '**.md' | |||
- '**.png' | |||
pull_request: | |||
paths-ignore: | |||
- '**.md' | |||
- '**.png' | |||
schedule: | |||
- cron: '29 7 * * 1' | |||
jobs: | |||
test: | |||
runs-on: ubuntu-latest | |||
strategy: | |||
fail-fast: false | |||
matrix: | |||
version: | |||
- master | |||
- 5.8 | |||
- 5.7.1 | |||
- 5.7 | |||
- 5.6.2 | |||
- 5.6.1 | |||
- 5.6 | |||
- 5.5.1 | |||
- 5.5 | |||
- 5.4.2 | |||
- 5.4.1 | |||
- 5.4 | |||
- 5.3.1 | |||
- 5.3 | |||
- 5.2 | |||
- 5.1.1 | |||
- 5.1 | |||
- 5.0.8 | |||
- 5.0.7 | |||
- 5.0.6 | |||
- 5.0.5 | |||
- 5.0.4 | |||
- 5.0.3 | |||
- 5.0.2 | |||
- 5.0.1 | |||
- 5.0.0 | |||
- 4.3.17 | |||
- 4.3.16 | |||
- 4.3.15 | |||
- 4.3.14 | |||
- 4.3.13 | |||
- 4.3.12 | |||
- 4.3.11 | |||
container: | |||
image: zshusers/zsh:${{ matrix.version }} | |||
steps: | |||
- uses: actions/checkout@v2 | |||
- run: install_packages bsdmainutils make procps | |||
- run: make test | |||
notify: | |||
runs-on: ubuntu-latest | |||
needs: test | |||
if: failure() && (github.repository_owner == 'zsh-users') | |||
steps: | |||
- | |||
name: Notify IRC | |||
uses: Gottox/irc-message-action@v1 | |||
with: | |||
server: irc.libera.chat | |||
channel: '#zsh-syntax-highlighting' | |||
nickname: zsyh-gh-bot | |||
message: '${{ github.ref }} failed tests: https://github.com/${{ github.repository }}/actions/runs/${{ github.run_id }}' |
@ -1,3 +1,24 @@ | |||
*.zwc* | |||
.pc/ | |||
docs/all.md | |||
current_theme.zsh | |||
secondary_theme.zsh | |||
theme_overlay.zsh | |||
*.txt | |||
test/out.parse | |||
test/res | |||
hold/* | |||
*.zwc | |||
### Vim | |||
# Swap | |||
[._]*.s[a-v][a-z] | |||
[._]*.sw[a-p] | |||
[._]s[a-v][a-z] | |||
[._]sw[a-p] | |||
# Session | |||
Session.vim | |||
# Temporary | |||
.netrwhist | |||
*~ | |||
# Auto-generated tag files | |||
tags |
@ -1 +0,0 @@ | |||
$Format:%H$ |
@ -0,0 +1,13 @@ | |||
addons: | |||
apt: | |||
packages: | |||
zsh | |||
install: | |||
- mkdir .bin | |||
- curl -L https://github.com/zunit-zsh/zunit/releases/download/v0.8.2/zunit > .bin/zunit | |||
- curl -L https://raw.githubusercontent.com/molovo/revolver/master/revolver > .bin/revolver | |||
- curl -L https://raw.githubusercontent.com/molovo/color/master/color.zsh > .bin/color | |||
before_script: | |||
- chmod u+x .bin/{color,revolver,zunit} | |||
- export PATH="$PWD/.bin:$PATH" | |||
script: zunit |
@ -1 +0,0 @@ | |||
0.8.0-alpha2-dev |
@ -0,0 +1,8 @@ | |||
tap: false | |||
directories: | |||
tests: tests | |||
output: tests/_output | |||
support: tests/_support | |||
time_limit: 0 | |||
fail_fast: false | |||
allow_risky: false |
@ -0,0 +1,144 @@ | |||
# News On Updates in F-Sy-H | |||
**2018-08-09** | |||
Added ideal string highlighting – FSH now handles any legal quoting and combination of `"`,`'` and `\` when | |||
highlighting program arguments. See the introduction for an example (item #14). | |||
**2018-08-02** | |||
Global aliases are now supported: | |||
 | |||
**2018-08-01** | |||
Hint – how to customize styles when using Zplugin and turbo mode: | |||
```zsh | |||
zplugin ice wait"1" atload"set_fast_theme" | |||
zplugin light zdharma/fast-syntax-highlighting | |||
set_fast_theme() { | |||
FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}paired-bracket]='bg=blue' | |||
FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}bracket-level-1]='fg=red,bold' | |||
FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}bracket-level-2]='fg=magenta,bold' | |||
FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}bracket-level-3]='fg=cyan,bold' | |||
} | |||
``` | |||
If you have set theme before an update of styles (e.g. recent addition of bracket highlighting) | |||
then please repeat `fast-theme {theme}` call to regenerate theme files. (**2018-08-09**: FSH | |||
now has full user-theme support, refer to [appropriate section of README](#customization)). | |||
**2018-07-30** | |||
Ideal highlighting of brackets (pairing, etc.) – no quoting can disturb the result: | |||
 | |||
`FAST_HIGHLIGHT[use_brackets]=1` to enable this feature (**2018-07-31**: not needed anymore, this highlighting is active by default). | |||
**2018-07-21** | |||
Chroma architecture now supports aliases. You can have `alias mygit="git commit"` and when `mygit` | |||
will be invoked everything will work as expected (Git chroma will be ran). | |||
**2018-07-11** | |||
There were problems with Ctrl-C not working when using FSH. After many days I've found a fix | |||
for this, it's pushed to master. | |||
Second, asynchronous path checking (useful on e.g. slow network drives, or when there are many files in directory) | |||
is now optional. Set `FAST_HIGHLIGHT[use_async]=1` to enable it. This saves some users from Zshell crashes | |||
– there's an unknown bug in Zsh. | |||
**2018-06-09** | |||
New chroma functions: `awk`, `make`, `perl`, `vim`. Checkout the [video](https://asciinema.org/a/186234), | |||
it shows functionality of `awk` – compiling of code and NOT running it. Perl can do this too: | |||
[video](https://asciinema.org/a/186098). | |||
**2018-06-06** | |||
FSH gained a new architecture – "chroma functions". They are similar to "completion functions", i.e. they | |||
are defined **per-command**, but instead of completing that command, they colorize it. Two chroma exist, | |||
for `Git` ([video](https://asciinema.org/a/185707), [video](https://asciinema.org/a/185811)) and for `grep` | |||
([video](https://asciinema.org/a/185942)). Checkout | |||
[example chroma](https://github.com/zdharma/fast-syntax-highlighting/blob/master/chroma/-example.ch) if you | |||
would like to highlight a command. | |||
 | |||
**2018-06-01** | |||
Highlighting of command substitution (i.e. `$(...)`) with alternate theme – two themes at once! It was just white before: | |||
 | |||
To select which theme to use for `$(...)` set the key `secondary=` in [theme ini file](https://github.com/zdharma/fast-syntax-highlighting/blob/master/themes/free.ini#L7). | |||
All shipped themes have this key set (only the `default` theme doesn't use second theme). | |||
Also added correct highlighting of descriptor-variables passed to `exec`: | |||
 | |||
**2018-05-30** | |||
For-loop is highlighted, it has separate settings in [theme file](https://github.com/zdharma/fast-syntax-highlighting/blob/master/themes/free.ini). | |||
 | |||
**2018-05-27** | |||
Added support for 256-color themes. There are six themes shipped with FSH. The command to | |||
switch theme is `fast-theme {theme-name}`, it has a completion which lists available themes | |||
and options. Checkout [asciinema recording](https://asciinema.org/a/183814) that presents | |||
the themes. | |||
**2018-05-25** | |||
Hash holding paths that shouldn't be grepped (globbed) – blacklist for slow disks, mounts, etc.: | |||
```zsh | |||
typeset -gA FAST_BLIST_PATTERNS | |||
FAST_BLIST_PATTERNS[/mount/nfs1/*]=1 | |||
FAST_BLIST_PATTERNS[/mount/disk2/*]=1 | |||
``` | |||
**2018-05-23** | |||
Assign colorizing now spans to variables defined by `typeset`, `export`, `local`, etc.: | |||
 | |||
Also, `zcalc` has a separate math mode and specialized highlighting – no more light-red colors because of | |||
treating `zcalc` like a regular command-line: | |||
 | |||
**2018-05-22** | |||
Array assignments were still boring, so I throwed in bracked colorizing: | |||
 | |||
**2018-05-22**<a name="assign-update"></a> | |||
Assignments are no more one-colour default-white. When used in assignment, highlighted are: | |||
- variables (outside strings), | |||
- strings (double-quoted and single-quoted), | |||
- math-mode (`val=$(( ... ))`). | |||
 | |||
**2018-01-06** | |||
Math mode is highlighted – expressions `(( ... ))` and `$(( ... ))`. Empty variables are colorized as red. | |||
There are 3 style names (fields of | |||
[FAST_HIGHLIGHT_STYLES](https://github.com/zdharma/fast-syntax-highlighting/blob/master/fast-highlight#L34) | |||
hash) for math-variable, number and empty variable (error): `mathvar`, `mathnum`, `matherr`. You can set | |||
them (like the animation below shows) to change colors. | |||
 |
@ -0,0 +1,166 @@ | |||
# Chroma Guide for F-Sy-H | |||
## Motivation | |||
Someone might want to create a detailed highlighting for a **specific program** | |||
and this document helps achieving this. It explains how chroma functions – the | |||
code behind such detailed highlighting – are constructed and used. | |||
## Keywords | |||
- `chroma` - a shorthand for `chroma function` – the thing that literally colorizes selected commands, like `git`, `grep`, etc. invocations, see `chroma function` below, | |||
- `big loop` - main highlighting code, a loop over tokens and at least 2 large structular constructs (big `if` and `case`); | |||
it is advanced, e.g. parses `case` statements, here-string, it basically constitutes 90% of the F-Sy-H project, | |||
- `chroma function` - a plugin-function that is called when a specific command occurs (e.g. when user enters `git` at | |||
command line) suppressing activity of `big loop` (i.e. no standard highlighting unless requested), | |||
- `token` - result of splitting whole command line (i.e. `$BUFFER`, the Zle variable) into bits called tokens, which are | |||
words in general, separated by spaces on the command line. | |||
## Overview Of Functioning | |||
1. Big loop is working – token by token processes command line, changes states (e.g. enters state "inside case | |||
statement") and in the end decides on color of the token currently processed. | |||
2. Big loop occurs a command that has a chroma, e.g. `git`. | |||
3. Big loop enters "chroma" state, calls associated chroma function. | |||
4. Chroma takes care of "chroma" state, ensures it will be set also for next token. | |||
5. "chroma" state is active, so all following tokens are routed to the chroma (in general skipping big-loop, see next items), | |||
6. When processing of a single token is complete, the associated chroma returns 0 | |||
(shell-truth) to request no further processing by the big loop. | |||
7. It can also return 1 so that single, current token will be passed into big-loop | |||
for processing (to do a standard highlighting). | |||
## Chroma-Function Arguments | |||
- `$1` - 0 or 1, denoting if it's the first call to the chroma, or a following one, | |||
- `$2` - the current token, also accessible by `$\__arg` from the upper scope - | |||
basically a private copy of `$__arg`; the token can be eg.: "grep", | |||
- `$3` - a private copy of `$_start_pos`, i.e. the position of the token in the | |||
command line buffer, used to add region_highlight entry (see man), | |||
because Zsh colorizes by *ranges* applied onto command line buffer (e.g. | |||
`from-10 to-13 fg=red`), | |||
- `$4` - a private copy of `$_end_pos` from the upper scope; denotes where current token | |||
ends (at which index in the string being the command line). | |||
So example invocation could look like this: | |||
---- | |||
chroma/-example.ch 1 "grep" "$_start_pos" "$_end_pos" | |||
---- | |||
Big-loop will be doing such calls for the user, after occurring a specific chroma-enabled command (like e.g. `awk`), and then until chroma will detect end of this chroma-enabled command (end of whole invocation, with arguments, etc.; in other words, when e.g. new line or `;`-character occurs, etc.). | |||
## Example Chroma-Function | |||
[source,zsh] | |||
---- | |||
# -*- mode: sh; sh-indentation: 4; indent-tabs-mode: nil; sh-basic-offset: 4; -*- | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
# | |||
# Example chroma function. It colorizes first two arguments as `builtin' style, | |||
# third and following arguments as `globbing' style. First two arguments may | |||
# be "strings", they will be passed through to normal higlighter (by returning 1). | |||
# | |||
# $1 - 0 or 1, denoting if it's first call to the chroma, or following one | |||
# | |||
# $2 - like above document says | |||
# | |||
# $3 - ... | |||
# | |||
# $4 - ... | |||
# | |||
# Other tips are: | |||
# - $CURSOR holds cursor position | |||
# - $BUFFER holds whole command line buffer | |||
# - $LBUFFER holds command line buffer that is left from the cursor, i.e. it's a | |||
# BUFFER substring 1 .. $CURSOR | |||
# - $RBUFFER is the same as LBUFFER but holds part of BUFFER right to the cursor | |||
# | |||
# The function receives $BUFFER but via sequence of tokens, which are shell words, | |||
# e.g. "a b c" is a shell word, while a b c are 3 shell words. | |||
# | |||
# FAST_HIGHLIGHT is a friendly hash array which allows to store strings without | |||
# creating global parameters (variables). If you need hash, go ahead and use it, | |||
# declaring first, under some distinct name like: typeset -gA CHROMA_EXPLE_DICT. | |||
# Remember to reset the hash and others at __first_call == 1, so that you have | |||
# a fresh state for new command. | |||
# Keep chroma-takever state meaning: until ;, handle highlighting via chroma. | |||
# So the below 8192 assignment takes care that next token will be routed to chroma. | |||
(( next_word = 2 | 8192 )) | |||
local __first_call="$1" __wrd="$2" __start_pos="$3" __end_pos="$4" | |||
local __style | |||
integer __idx1 __idx2 | |||
(( __first_call )) && { | |||
# Called for the first time - new command. | |||
# FAST_HIGHLIGHT is used because it survives between calls, and | |||
# allows to use a single global hash only, instead of multiple | |||
# global string variables. | |||
FAST_HIGHLIGHT[chroma-example-counter]=0 | |||
# Set style for region_highlight entry. It is used below in | |||
# '[[ -n "$__style" ]] ...' line, which adds highlight entry, | |||
# like "10 12 fg=green", through `reply' array. | |||
# | |||
# Could check if command `example' exists and set `unknown-token' | |||
# style instead of `command' | |||
__style=${FAST_THEME_NAME}command | |||
} || { | |||
# Following call, i.e. not the first one | |||
# Check if chroma should end – test if token is of type | |||
# "starts new command", if so pass-through – chroma ends | |||
[[ "$__arg_type" = 3 ]] && return 2 | |||
if [[ "$__wrd" = -* ]]; then | |||
# Detected option, add style for it. | |||
[[ "$__wrd" = --* ]] && __style=${FAST_THEME_NAME}double-hyphen-option || \ | |||
__style=${FAST_THEME_NAME}single-hyphen-option | |||
else | |||
# Count non-option tokens | |||
(( FAST_HIGHLIGHT[chroma-example-counter] += 1, __idx1 = FAST_HIGHLIGHT[chroma-example-counter] )) | |||
# Colorize 1..2 as builtin, 3.. as glob | |||
if (( FAST_HIGHLIGHT[chroma-example-counter] <= 2 )); then | |||
if [[ "$__wrd" = \"* ]]; then | |||
# Pass through, fsh main code will do the highlight! | |||
return 1 | |||
else | |||
__style=${FAST_THEME_NAME}builtin | |||
fi | |||
else | |||
__style=${FAST_THEME_NAME}globbing | |||
fi | |||
fi | |||
} | |||
# Add region_highlight entry (via `reply' array). | |||
# If 1 will be added to __start_pos, this will highlight "oken". | |||
# If 1 will be subtracted from __end_pos, this will highlight "toke". | |||
# $PREBUFFER is for specific situations when users does command \<ENTER> | |||
# i.e. when multi-line command using backslash is entered. | |||
# | |||
# This is a common place of adding such entry, but any above code can do | |||
# it itself (and it does in other chromas) and skip setting __style to | |||
# this way disable this code. | |||
[[ -n "$__style" ]] && (( __start=__start_pos-${#PREBUFFER}, __end=__end_pos-${#PREBUFFER}, __start >= 0 )) && reply+=("$__start $__end ${FAST_HIGHLIGHT_STYLES[$__style]}") | |||
# We aren't passing-through, do obligatory things ourselves. | |||
# _start_pos=$_end_pos advainces pointers in command line buffer. | |||
(( this_word = next_word )) | |||
_start_pos=$_end_pos | |||
return 0 | |||
---- | |||
@ -0,0 +1,441 @@ | |||
<!-- START doctoc generated TOC please keep comment here to allow auto update --> | |||
<!-- DON'T EDIT THIS SECTION, INSTEAD RE-RUN doctoc TO UPDATE --> | |||
**Table of Contents** *generated with [DocToc](https://github.com/thlorenz/doctoc)* | |||
- [2018-08-14, received $30](#2018-08-14-received-30) | |||
- [2018-08-03, received $8](#2018-08-03-received-8) | |||
- [2018-08-02, received $3 from Patreon](#2018-08-02-received-3-from-patreon) | |||
- [2018-07-31, received $7](#2018-07-31-received-7) | |||
- [2018-07-28, received $2](#2018-07-28-received-2) | |||
- [2018-07-25, received $3](#2018-07-25-received-3) | |||
- [2018-07-20, received $3](#2018-07-20-received-3) | |||
- [2018-06-17, received ~$155 (200 CAD)](#2018-06-17-received-155-200-cad) | |||
- [2018-06-10, received $10](#2018-06-10-received-10) | |||
- [2018-05-25, received $50](#2018-05-25-received-50) | |||
<!-- END doctoc generated TOC please keep comment here to allow auto update --> | |||
Below are reports about what is being done with donations, i.e. which commits | |||
are created thanks to them, which new features are added, etc. From the money | |||
I receive I buy myself coffee and organize the time to work on the requested | |||
features, sometimes many days in a row. | |||
## 2018-08-14, received $30 | |||
* **Project**: **[Zplugin](https://github.com/zdharma/zplugin)** | |||
* **Goal**: Create a binary Zsh module with one Zplugin optimization and optionally some | |||
other features. | |||
* **Status**: The job is done. | |||
Thanks to this donation I have finally started to code **[binary Zplugin module]( | |||
https://github.com/zdharma/zplugin#quick-start-module-only)**, which is a big step onward | |||
in evolution of Zplugin. I've implemented and published the module with 3 complete | |||
features: 1) `load` optimization, 2) autocompilation of scripts, 3) profiling of script | |||
load times. | |||
Commit list: | |||
``` | |||
2018-08-22 7b96fad doc: mod-install.sh | |||
2018-08-22 ba1ba64 module: Update zpmod usage text | |||
2018-08-22 b0d72e8 zplugin,*autoload: `module' command, manages new zdharma/zplugin module | |||
2018-08-22 706bbb3 Update Zsh source files to latest | |||
2018-08-20 b77426f module: source-study builds report with milliseconds without fractions | |||
2018-08-20 c3cc09b module: Updated zpmod_usage, i.a. with `source-study' sub-command | |||
2018-08-20 6190295 module: Go back to subcommand-interface to `zpmod'; simple option parser | |||
2018-08-20 881005f module: Report on sourcing times is shown on `zpmod -S`. Done generation | |||
2018-08-19 e5d046a module: Correct conditions on zwc file vs. script file (after stats) | |||
2018-08-19 1282c21 module: Duration of sourcing a file is measured and stored into a hash | |||
2018-08-18 e080153 module: Overload both `source' and `.' builtins | |||
2018-08-18 580efb8 module: Invoke bin_zcompile with -U option (i.e. no alias expansion) | |||
2018-08-18 b7d9836 module: Custom `source' ensures script is compiled, compiles if not | |||
2018-08-18 1e75a47 module: Code cleanup, vim folding | |||
2018-08-18 a4a02f3 module: Finally working `source'/`.' overload (used options translating) | |||
2018-08-16 99bba56 module: zpmod_usage gained content | |||
2018-08-16 04703cd module: Add the main builtin zpmod with report-append which is working | |||
2018-08-16 cd6dc19 module: my_ztrdup_glen, zp_unmetafy_zalloc | |||
2018-08-16 6d44e36 module: Cleanup, `source' overload after patron leoj3n restarted module | |||
``` | |||
## 2018-08-03, received $8 | |||
* **Project**: **[zdharma/history-search-multi-word](https://github.com/zdharma/history-search-multi-word)** | |||
* **Goal**: Allow calling `zle reset-prompt` (Zshell feature). | |||
* **Status**: The job is done. | |||
A user wanted to be able to call `reset-prompt` Zshell widget without disturbing my project | |||
`history-search-multi-word`. I've implemented the necessary changes to HSMW. | |||
Commit list: | |||
``` | |||
2018-08-04 9745d3d hsmw: reset-prompt-protect zstyle – allow users to run zle reset-prompt | |||
2018-08-04 ce48a53 hsmw: More typo-like lackings of % substitution | |||
2018-08-04 7e2d79b hsmw: A somewhat typo, missing % substitution | |||
``` | |||
## 2018-08-02, received $3 from Patreon | |||
* **Project**: **[zdharma/fast-syntax-highlighting](https://github.com/zdharma/fast-syntax-highlighting)** | |||
* **Goal**: No goal set up. | |||
* **Status**: Bug-fixing work. | |||
I did bug-fixing run on `fast-syntax-highlighting`, spotted many small and sometimes important things to | |||
improve. Did one bigger thing – added global-aliases functionality. | |||
Commit list: | |||
``` | |||
2018-08-02 1e854f5 -autoload.ch: Don't check existence for arguments that are variables | |||
2018-08-02 14cdc5e *-string-*: Support highlighter cooperation in presence of $PREBUFFER | |||
2018-08-02 2d8f0e4 *-highlight: Correctly highlight $VAR, $~VAR, ${+VAR}, etc. in strings | |||
2018-08-02 e3032d9 *-highlight: ${#PREBUFFER} -> __PBUFLEN, equal performance | |||
2018-08-02 f0a7121 *-highlight: Make case conditions and brackets highlighter compatible | |||
2018-08-02 781f68e *-highlight: Recognize more case-item-end tokens | |||
2018-08-02 206c122 *-highlight: Remove unused 4th __arg_type | |||
2018-08-02 c6da477 *-string-*: Handle 'abc\' – no slash-quoting here. Full quoting support | |||
2018-08-02 52e0176 *-string-*: Fix bug, third level was getting wrong style | |||
2018-08-02 5edbfae -git.ch: Support "--message=..." syntax (commit) | |||
2018-08-02 669d4b7 -git.ch: Handle "--" argument (stops options) | |||
2018-08-02 4fae1f2 -make.ch: Handle make's -f option | |||
2018-08-02 3fd32fe -make.ch: Handle make's -C option | |||
2018-08-02 31751f5 -make.ch: Recognize options that obtain argument | |||
2018-08-02 e480f18 -make.ch: Fix reply-var clash, gained consistency | |||
2018-08-02 0e8bc1e Updated README.md | |||
2018-08-02 eee0034 images: global-alias.png | |||
2018-08-02 00b41ef *-highlight,themes,fast-theme: Support for global aliases #41 | |||
``` | |||
## 2018-07-31, received $7 | |||
* **Project**: **[zdharma/fast-syntax-highlighting](https://github.com/zdharma/fast-syntax-highlighting)** | |||
* **Goal**: Implement ideal brackets highlighting. | |||
* **Status**: The job is done. | |||
When a source code is edited e.g. in `Notepad++` or some IDE, then most often brackets are somehow matched to | |||
each other, so that the programmer can detect mistakes. `Fast-syntax-highlighting` too gained that feature. It | |||
was done in such a way that FSH cannot make any mistake, colors will perfectly match brackets to each other. | |||
Commit list: | |||
``` | |||
2018-07-31 2889860 *-highlight: Correct place to initialize $_FAST_COMPLEX_BRACKETS | |||
2018-07-31 2bde2a9 Performance status -15/8/8 | |||
2018-07-31 5078261 *-highlight,README: Brackets highlighter active by default | |||
2018-07-31 2ee3073 *-highlight,*string-*: Brackets in [[..]], ((..)), etc. handled normally | |||
2018-07-31 776b12d plugin.zsh: $_ZSH_HIGHLIGHT_MAIN_CACHE -> $_FAST_MAIN_CACHE | |||
2018-07-30 2867712 plugin.zsh: Fix array parameter created without declaring #43 | |||
2018-07-30 cbe5fc8 Updated README.md | |||
2018-07-30 2bd3291 images: brackets.gif | |||
2018-07-30 ef23a96 *-string-*: Bug-fix, correctly use theme styles | |||
2018-07-30 9046f82 plugin.zsh: Attach the new brackets highlighter; F_H[use_brackets]=1 | |||
2018-07-30 b33a5fd fast-theme: Support 4 new styles (for brackets) | |||
2018-07-30 a03f004 themes: Add 4 new styles (brackets) | |||
2018-07-30 2448cdc *-string-*: Additional highlight of bracket under cursor; more styles | |||
2018-07-30 5e1795e *-string-*: Highlighter for brackets, handles all quotings; detached | |||
``` | |||
## 2018-07-28, received $2 | |||
* **Project**: **[zdharma/fast-syntax-highlighting](https://github.com/zdharma/fast-syntax-highlighting)** | |||
* **Goal**: Distinguish file and directory when highlighting | |||
* **Status**: The job is done. | |||
A user requested that when `fast-syntax-highlighting` colorizes the command line it should use different | |||
styles (e.g. colors) for token that's a *file* and that's a *directory*. It was a reasonable idea and I've | |||
implemented it. | |||
Commit list: | |||
``` | |||
2018-07-28 7f48e04 themes: Extend all themes with new style `path-to-dir' | |||
2018-07-28 c7c6a91 fast-theme: Support for new style `path-to-dir' | |||
2018-07-28 264676c *-highlight: Differentiate path and to-dir path. New style: path-to-dir | |||
``` | |||
## 2018-07-25, received $3 | |||
* **Project**: **[zdharma/zshelldoc](https://github.com/zdharma/zshelldoc)** | |||
* **Goal**: Implement documenting of used environment variables. | |||
* **Status**: The job is done. | |||
Zshelldoc generates code-documentation like Doxygen or Javadoc, etc. User requested a | |||
new feature: the generated docs should enumerate environment variables used and/or | |||
exported by every function. Everything went fine and this feature has been implemented. | |||
Commit list: | |||
``` | |||
2018-07-26 f63ea25 Updated README.md | |||
2018-07-26 3af0cf7 *detect: Get `var' from ${var:-...} and ${...:+${var}} and other subst | |||
2018-07-25 2932510 *adoc: Better language in output document (about exported vars) #5 | |||
2018-07-25 f858dd8 *adoc: Include (in the output document) data on env-vars used #5 | |||
2018-07-25 80e3763 *adoc: Include data on exports (environment) in the output document #5 | |||
2018-07-25 ca576e2 *detect: Detect which env-vars are used, store meta-data in data/ #5 | |||
2018-07-25 f369dcc *detect: Function `find-variables' reported "$" as a variable, fixed #5 | |||
2018-07-25 e243dab *detect: Function `find-variables' #5 | |||
2018-07-25 5b34bb1 *transform: Detect exports done by function/script-body, store #5 | |||
``` | |||
## 2018-07-20, received $3 | |||
* **Project**: **[zdharma/zshelldoc](https://github.com/zdharma/zshelldoc)** | |||
* **Goal**: Implement stripping of leading `#` char from functions' descriptions. | |||
* **Status**: The job is done. | |||
A user didn't like that functions' descriptions in the JavaDoc-like document (generated with Zshelldoc) all | |||
contain a leading `#` character. I've added stripping of this character (it is there in the processed source | |||
code) controlled by a new Zshelldoc option. | |||
Commit list: | |||
``` | |||
2018-07-20 172c220 zsd,*adoc,README: Option --scomm to strip "#" from function descriptions | |||
``` | |||
## 2018-06-17, received ~$155 (200 CAD) | |||
* **Project**: **[zdharma/fast-syntax-highlighting](https://github.com/zdharma/fast-syntax-highlighting)** | |||
* **Goal**: No goal set up. | |||
* **Status**: Done intense research. | |||
I've created 2 new branches: `Hue-optimization` (33 commits) and `Tidbits-feature` (22 commits). Those were | |||
branches with architectural changes and extraordinary features. The changes yielded to be too slow, and I had | |||
to withdraw the merge. Below are fixing and optimizing commits (i.e. the valuable ones) that I've restored | |||
from the two branches into master. | |||
Commit list: | |||
``` | |||
2018-07-21 dab6576 *-highlight: Merge-restore: remove old comments | |||
2018-07-21 637521f *-highlight: Merge-restore: a threshold on # of zle .redisplay calls | |||
2018-07-21 4163d4d *-highlight: Merge-restore: optimize four $__arg[1] = ... cases | |||
2018-07-21 0f01195 *-highlight: Merge-restore: can remove one (Q) dequoting | |||
2018-07-21 39a4ec6 *-highlight: Merge-restore: $v = A* is faster than $v[1] = A, tests: | |||
2018-07-21 99d6b33 *-highlight: Merge-restore: optimize-out ${var:1} Bash syntax | |||
2018-07-21 719c092 *-highlight: Merge-restore: allow $V/cmd, "$V/cmd, "$V/cmd", "${V}/cmd" | |||
2018-07-21 026941d *-highlight: Merge-restore: stack pop in single instruction, not two | |||
2018-07-21 3467e3d *-highlight: Merge-restore: more reasonable redirection-detecting code | |||
2018-07-21 00d25ee *-highlight: Merge-restore: one active_command="$__arg" not needed (?) | |||
2018-07-21 1daa6b3 *-highlight: Merge-restore: simplify ; and \n code short-paths | |||
2018-07-21 55d65be *-highlight: Merge-restore: proc_buf advancement via patterns (not (i)) | |||
2018-07-21 cc55546 *-highlight: Merge-restore: pattern matching to replace (i) flag | |||
``` | |||
## 2018-06-10, received $10 | |||
* **Project**: **[zdharma/fast-syntax-highlighting](https://github.com/zdharma/fast-syntax-highlighting)** | |||
* **Goal**: No goal set up. | |||
* **Status**: Done intense experimenting. | |||
I was working on *chromas* – command-specific colorization. I've added `which` and | |||
`printf` colorization, then added asynchronous path checking (needed on slow network | |||
drives), then coded experimental `ZPath` feature for chromas, but it couldn't be optimized | |||
so I had to resign of it. | |||
Commit list: | |||
``` | |||
2018-06-12 c4ed1c6 Optimization – the same idea as in previous patch, better method | |||
2018-06-12 c36feef Optimization – a) don't index large buffer, b) with negative index | |||
2018-06-12 2f03829 Performance status 2298 / 1850 | |||
2018-06-12 14f5159 New working feature – ZPath. It requires optimization | |||
2018-06-12 e027c40 -which.ch: One of commands can apparently return via stderr (#27) | |||
2018-06-11 5b8004f New chroma `ruby', works like chroma `perl', checks syntax via -ce opts | |||
2018-06-10 ca2e18b *-highlight: Async path checking has now 8-second cache | |||
2018-06-10 e071469 *-highlight: Remove path-exists queue clearing | |||
2018-06-10 5a6684c *-highlight: Support for asynchronous path checking | |||
2018-06-10 1d7d6f5 New chroma: `printf', highlights special sequences like %s, %20s, etc. | |||
2018-06-10 8f59868 -which.ch: Update main comment on purpose of this chroma | |||
2018-06-10 5f4ece2 -which.ch: Added `whatis', it has only 1st line if output used | |||
2018-06-10 e2d173e -which.ch: Uplift: handle `which' called on a function, /usr/bin/which | |||
``` | |||
## 2018-05-25, received $50 | |||
* **Project**: **[zdharma/fast-syntax-highlighting](https://github.com/zdharma/fast-syntax-highlighting)** | |||
* **Goal**: No goal set up. | |||
* **Status**: New ideas and features. | |||
I was working from May, 25 to June, 9 and came up with key ideas and implemented them. First were *themes* | |||
that were very special because they were using `INI` files instead of some Zsh-script format. Creating themes | |||
for `fast-syntax-highlighting` is thus easy and fun. Then I came up with *chromas*, command-specific | |||
highlighting, which redefine how syntax-highlighting for Zshell works – detailed highlighting for e.g. Git | |||
became possible, the user is informed about e.g. a mistake even before running a command. Overall 178 commits | |||
in 16 days. | |||
``` | |||
2018-06-09 3f72e6c -git.ch: `revert' works almost like `checkout', attach `revert' there | |||
2018-06-09 b892743 Updated CHROMA_GUIDE.adoc | |||
2018-06-09 f05643d Revert "Revert "Updated CHROMA_GUIDE.md"" | |||
2018-06-09 729bf7f Revert "Revert "CHROMA_GUIDE: Remove redundant comments, uplift"" | |||
2018-06-09 48a4e0c Revert "CHROMA_GUIDE: Remove redundant comments, uplift" | |||
2018-06-09 55ede0a Revert "Updated CHROMA_GUIDE.md" | |||
2018-06-09 17a28ba New chroma `-docker.ch' that verifies image ID passed to `image rm' | |||
2018-06-09 868812a -make.ch,*-make-targets: Check Makefile exists, use 7 second cache, #24 | |||
2018-06-09 73df278 -sh.ch: Attach fish, has -c option, though different syntax, let's try | |||
2018-06-09 3a73b8e Updated CHROMA_GUIDE.md | |||
2018-06-09 29d04c8 CHROMA_GUIDE: Remove redundant comments, uplift | |||
2018-06-09 22ce1d8 -sh.ch,*-highlight: Attach to 2 other shells, Zsh and Bash | |||
2018-06-09 f54e44f New chroma `-sh.ch', colorizes code passed to `sh' with -c option | |||
2018-06-09 f5d2375 CHROMA_GUIDE: Add example code block (rendered broken in mdown) | |||
2018-06-09 08f4b28 CHROMA_GUIDE: Switch to asciidoc (rename) | |||
2018-06-09 4e03609 CHROMA_GUIDE.md | |||
2018-06-09 bbcf2d6 -source.ch: Word "source" should be highlighted as builtin | |||
2018-06-09 6739b8b New chroma – `source' to handle . and source builtins | |||
2018-06-09 b961211 gitignore: ignore more paths | |||
2018-06-09 59d5d09 Updated README.md | |||
2018-06-09 f6d4d19 Updated README.md | |||
2018-06-09 eb31324 Update README.md (figlet logo) | |||
2018-06-09 71dcc5f Performance status 298 / 479 | |||
2018-06-09 00c5f8f *-highlight: Add comments | |||
2018-06-09 232903c -awk.ch: Highlight `sub' function, not working {, } highlighting | |||
2018-06-09 b5241ba *-highlight: Much better $( ) recursion, would say problems-free, maybe | |||
2018-06-08 6c69437 *-highlight: Larger buffer (110 -> 250) for $( ) matching | |||
2018-06-08 f2b7a96 -awk.ch: Syntax check code passed to awk. Awk is very forgiving, though | |||
2018-06-08 c53d8ba -vim.ch: Pass almost everything to big-loop, check if vim exists | |||
2018-06-08 7fbf7cd chroma: New chroma `vim', shows last opened files under prompt | |||
2018-06-08 06e4570 gitignore: Extend .gitignore | |||
2018-06-08 3184ba1 chroma: All chroma functions end chroma mode on e.g. | and similar | |||
2018-06-08 070077d *-highlight,-example.ch: Rename arg_type -> __arg_type, use it to end | |||
2018-06-08 6c2411e -awk.ch: Use the new theme style `subtle-bg' | |||
2018-06-08 9ec8d63 themes: All themes (remaining 4) to support `subtle-bg' style | |||
2018-06-08 66e848b fast-theme: New theme key `subtle-bg', default & clean.ini support it | |||
2018-06-08 1e794f9 -awk.ch: More keywords highlighted | |||
2018-06-08 f3bbaca -awk.ch: Don't highlight keywords when they only contain proper keyword | |||
2018-06-08 e4d5283 -awk.ch: Fix mistake (indices), was highlighting 1 extra trailing letter | |||
2018-06-08 eebbb19 -awk.ch: Initialize FSH_LIST | |||
2018-06-08 8ec24ca *-highlight: Missing math function for awk | |||
2018-06-08 d8e423a -awk.ch: Highlight more keywords, via more general code | |||
2018-06-07 ee26e66 Commit missing -fast-make-targets | |||
2018-06-07 9d4f2b5 New chroma `-awk.ch', colorizes regex characters and a keyword (print) | |||
2018-06-07 def5133 -example.ch: Add comments | |||
2018-06-07 f31a2d0 New chroma -make.ch, verifies if target is correct | |||
2018-06-07 623b8ce -perl.ch: Use correct keys in FAST_HIGHLIGHT hash | |||
2018-06-07 090f420 themes: Make all themes provide {in,}correct-subtle styles | |||
2018-06-07 2201fb6 New -perl.ch chroma, syntax-checks perl code; 2 new theme entries | |||
2018-06-06 4b9598e *-highlight: Fix bug in math highlight – allow variables starting with _ | |||
2018-06-06 708afec *-highlight: Fix FAST_BLIST_PATTERNS not expanding path to absolute one | |||
2018-06-06 caef05a -example.ch: Update, fix typos, remove unused code | |||
2018-06-06 3fb192a Updated README.md | |||
2018-06-06 6de0c82 images: git_chroma.png | |||
2018-06-06 2852fdd -grep.ch (new): Special highlighting for grep – -grep.ch chroma function | |||
2018-06-06 f216785 -example.ch: Added comments | |||
2018-06-06 4ab5b36 -example.ch: Add comments | |||
2018-06-06 380cd12 -example.ch: Added comments | |||
2018-06-06 c8947cc -example.ch: Add comments | |||
2018-06-06 f2e273e -example.ch: Add comments | |||
2018-06-06 2f3565b plugin.zsh: Fix parse error | |||
2018-06-06 4f1a9bd plugin.zsh: Added $fpath handling, to match what README contains | |||
2018-06-06 cc9adb5 -example.ch: Change and extend comments | |||
2018-06-06 3128fff -git.ch: More intelligent `checkout' highlighting – ref is first | |||
2018-06-06 4b6f54b -git.ch: Support for `checkout' subcommand | |||
2018-06-06 1930d37 -example.ch: Added example chroma function | |||
2018-06-05 d79cd85 -git.ch: Add comments | |||
2018-06-05 1473c9e -git.ch: Add comments | |||
2018-06-05 0cb1419 -git.ch: Message passed after -m is checked for the 72 chars boundary | |||
2018-06-05 3f99944 -git.ch: Architectural uplift of git chroma | |||
2018-06-05 e044d13 -git.ch: Single place to add entry to $reply (target: region_highlight) | |||
2018-06-05 3a84364 -git.ch: Handle quoted non-option arguments, also partly quoted: "abc | |||
2018-06-05 d635bf4 -fast-run-git-command, it handles cache automatically, decimates source | |||
2018-06-05 102ea78 -git.ch: Smart handling of `git push', remotes and branches are verified | |||
2018-06-04 be88850 Performance status [+] 39+77=116 / -26+24=-2 | |||
2018-06-04 0e033f8 Experimental chroma support, currently active only on command `git' | |||
2018-06-04 43ae221 *-highlight: Emacs mode-line | |||
2018-06-04 938ad29 test: New "-git" parsing option, test results, -git.ch included | |||
2018-06-04 e433fbc fast-theme: Explicitly return 0; added Emacs mode-line | |||
2018-06-04 66e9b3c *-highlight: Detection of $( ) now doesn't go for $(( )) as a candidate | |||
2018-06-04 488a580 chroma: Empty chroma function for `git' | |||
2018-06-04 f54d770 *-highlight: Rename $cur_cmd to $active_command | |||
2018-06-04 3f24e68 *-highlight: Make sudo and always-block compatible with `case' handling | |||
2018-06-02 cd82637 themes: forest.ini to support 3 new `case' styles | |||
2018-06-02 e1e993e themes: safari.ini & zdharma.ini to support 3 new `case' styles | |||
2018-06-02 2e78a02 themes: clean.ini & default.ini to support 3 new `case' styles | |||
2018-06-02 c1c3aab themes: free.ini to support 3 new `case' styles | |||
2018-06-02 70a7e18 fast-theme,*-highlight: 3 new styles for `case' higlighting | |||
2018-06-02 8d90dc2 *-highlight: Support for `case' highlighting | |||
2018-06-02 10d291c *-highlight: Softer state manipulation, less rigid =1 etc. assignments | |||
2018-06-02 6159507 *-highlight: Don't highlight closing ) with style `assign' | |||
2018-06-02 1fc2450 *-highlight: One complex math command optimization, top of the loop | |||
2018-06-02 cc49247 *-highlight: Fix improper state after assignment (command | regular) | |||
2018-06-02 02942b8 Updated README.md | |||
2018-06-02 5e28259 images: eval_cmp.png | |||
2018-06-02 df92fed *-highlight: Fix removal of trailing "/' when recursing in eval | |||
2018-06-02 4f61938 Performance status 46 / 44 | |||
2018-06-02 a5ade0e *-highlight: Recursive highlighting of eval string argument | |||
2018-06-02 e91847b *-highlight: Don't recurse when not at main *-process call | |||
2018-06-02 fca8603 *-highlight: Support assignments of arrays when key is taken from array | |||
2018-06-02 5d70f01 *-highlight: Math highlighting recognizes ${+VAR} | |||
2018-06-02 c48eb0d *-highlight: Math colorizing recognizes variables in braces ${HISTISZE} | |||
2018-06-02 53dd85a *-highlight: Allow -- for precommand modifiers command & exec | |||
2018-06-02 d9fe110 *-highlight: Detect globbing also when `##' occurs | |||
2018-06-02 55c923d Performance status 132 / 66 | |||
2018-06-02 3bd8f07 themes: safari.ini to have globbing color specifically selected | |||
2018-06-02 2b55260 themes: free.ini to have globbing color specifically selected | |||
2018-06-02 494868e themes: clean.ini to have globbing color specifically selected | |||
2018-06-01 fca6b3d images: herestring.png #9 | |||
2018-06-01 f9842c1 themes: forest.ini to use underline instead of bg color #9 | |||
2018-06-01 c25c539 themes: Small tune-up of forest & zdharma themes for here-string #9 | |||
2018-06-01 988d504 themes: Rudimentary (all same) configuration of here-string tokens #9 | |||
2018-06-01 99842d2 fast-theme,*-highlight: Support for here-string, can use bg color #9 | |||
2018-06-01 f739c30 Updated README.md | |||
2018-06-01 7fa8451 images: execfd.png execfd_cmp.png | |||
2018-06-01 d7384f1 themes: All themes gained `exec-descriptor=` key, now supported by code | |||
2018-06-01 d66d140 themes: Fix improper effect of s/red/.../ substitution in clean,forest | |||
2018-06-01 f7ee5e2 fast-theme,*-highlight: Support highlighting of {FD} etc. passed to exec | |||
2018-06-01 e5c5534 *-highlight: Proper states for precmd (command,exec) option handling | |||
2018-06-01 647b198 images: New cmdsubst.png | |||
2018-06-01 74bdc4c Updated README.md | |||
2018-06-01 86eb15e images: theme.png | |||
2018-06-01 5169e82 Updated README.md | |||
2018-06-01 1c462b7 Updated README.md | |||
2018-06-01 4c21da4 images: cmdsubst.png | |||
2018-06-01 b39996e *-highlight: Switch theme to secondary when descending into $() #15 | |||
2018-06-01 bf96045 themes: Equip all themes with key `secondary' (an alternate theme) #15 | |||
2018-06-01 aa1b112 fast-theme: Generate secondary theme (from key `secondary' in theme) #15 | |||
2018-06-01 6dd3bd3 *-highlight: Support for multiple active themes #15 | |||
2018-06-01 8a32944 *-highlight: Fix "$() found?" comparison | |||
2018-06-01 3651605 *-highlight: Significant change: the parser is called recursively on $() | |||
2018-05-31 882d88b test,*-highlight: New -ooo performance test; highlighter takes arguments | |||
2018-05-31 5ba1178 *-highlight: Optimization - compute __arg length once | |||
2018-05-30 b2a0126 *-highlight: Allow multiple separate options for `command', `exec' (#10) | |||
2018-05-30 5804e9a *-highlight: Correct state after option for precommand (#10) | |||
2018-05-30 1247b64 *-highlight: Simpler and more accurate option-testing for exec, command (#10) | |||
2018-05-30 d87fed4 *-highlight: Correctly highlight options for `command' and `exec' (#10) | |||
2018-05-30 8c3e75e *-highlight: Double-hyphen (--) stops option recognition and colorizing | |||
2018-05-30 1c5a56c *-highlight: Support ${VAR} at command position (not only $VAR) | |||
2018-05-30 f19d761 Updated README.md | |||
2018-05-30 4a27351 images: for-loop | |||
2018-05-30 4d650de themes: zdharma.ini to support for-loop | |||
2018-05-30 45cafbc themes: safari.ini to support for-loop | |||
2018-05-30 8bb9ee0 themes: free.ini to support for-loop | |||
2018-05-30 f25a059 themes: forest.ini to support for-loop | |||
2018-05-29 093d79e themes: default.ini to support for-loop | |||
2018-05-29 446cb7b clean.ini,fast-theme: Clean-theme & theme subsystem to support for-loop | |||
2018-05-29 1bb701f *-highlight: Move $variable highlighting from case to if-block | |||
2018-05-29 b8413e9 *-highlight: For-loop highlighting, working, needs few upgrades | |||
2018-05-28 7bec6e5 *-highlight: Three more simple vs. complex math operation optimizations | |||
2018-05-27 baae683 *-highlight: Optimise complex math command into single one with & and ~ | |||
2018-05-27 2dc3103 *-highlight: Optimise complex math command into single one with & and ~ | |||
2018-05-27 291f905 _fast-theme: Update -t/--test description | |||
2018-05-27 ec305f6 fast-theme: Help message treats about -t/--test | |||
2018-05-27 0e1d19a Updated README.md | |||
2018-05-27 5c3c911 Updated README.md | |||
2018-05-26 76af248 themes: A fix for zdharma theme, 61 -> 63, a lighter color for builtins | |||
2018-05-26 8eca0f2 *fast-theme: Ability to test theme after setting it (-t/--test) | |||
2018-05-26 d3a7922 *-highlight: Fix in_array_assignment setting when closing ) found | |||
2018-05-26 796c482 *-highlight: Make parameters' names exotic blank-var detection to work | |||
2018-05-26 ae3913f _fast-theme: Complete theme names | |||
2018-05-26 d212945 *-highlight,plugin.zsh,default.ini: Uplift of fg=112-fix code | |||
2018-05-26 ee56f65 *-highlight,plugin.zsh: Final fix for fg=112 assignment – use zstyle | |||
2018-05-26 391f5a4 fast-theme: Set `theme' zstyle in `:plugin:fast...' to given theme | |||
2018-05-26 e0dc086 plugin.zsh: Fix the fg=112 assignment done for `variable' style | |||
2018-05-26 17c9286 Updated README.md | |||
2018-05-26 4774c1c fast-theme: Add completion for this function | |||
2018-05-26 d971f39 fast-theme: Detect lack of theme name in arguments | |||
2018-05-26 74f0d4d fast-theme: Use standard option parsing (zparseopts) and typical options | |||
2018-05-26 d9c6180 New theme: `forest' | |||
2018-05-26 419c156 New theme: `zdharma' | |||
2018-05-26 a7735df gitignore | |||
2018-05-26 99db69a New theme: `free' | |||
2018-05-26 73619ff New theme: `clean' | |||
2018-05-25 52307fb Theme support, 1 extra theme – `safari' | |||
2018-05-25 41df55b *-highlight: (k) subscript flag is sufficient, no need for (K) | |||
2018-05-25 cb21c05 Updated README.md | |||
2018-05-25 a580cff *-highlight: FAST_BLIST_PATTERNS | |||
``` |
@ -1,99 +0,0 @@ | |||
Hacking on zsh-syntax-highlighting itself | |||
========================================= | |||
This document includes information for people working on z-sy-h itself: on the | |||
core driver (`zsh-syntax-highlighting.zsh`), on the highlighters in the | |||
distribution, and on the test suite. It does not target third-party | |||
highlighter authors (although they may find it an interesting read). | |||
The `main` highlighter | |||
---------------------- | |||
The following function `pz` is useful when working on the `main` highlighting: | |||
```zsh | |||
pq() { | |||
(( $#argv )) || return 0 | |||
print -r -l -- ${(qqqq)argv} | |||
} | |||
pz() { | |||
local arg | |||
for arg; do | |||
pq ${(z)arg} | |||
done | |||
} | |||
``` | |||
It prints, for each argument, its token breakdown, similar to how the main | |||
loop of the `main` highlighter sees it. | |||
Testing the `brackets` highlighter | |||
---------------------------------- | |||
Since the test harness empties `ZSH_HIGHLIGHT_STYLES` and the `brackets` | |||
highlighter interrogates `ZSH_HIGHLIGHT_STYLES` to determine how to highlight, | |||
tests must set the `bracket-level-#` keys themselves. For example: | |||
```zsh | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
BUFFER='echo ({x})' | |||
expected_region_highlight=( | |||
"6 6 bracket-level-1" # ( | |||
"7 7 bracket-level-2" # { | |||
"9 9 bracket-level-2" # } | |||
"10 10 bracket-level-1" # ) | |||
) | |||
``` | |||
Testing the `pattern` and `regexp` highlighters | |||
----------------------------------------------- | |||
Because the `pattern` and `regexp` highlighters modifies `region_highlight` | |||
directly instead of using `_zsh_highlight_add_highlight`, the test harness | |||
cannot get the `ZSH_HIGHLIGHT_STYLES` keys. Therefore, when writing tests, use | |||
the style itself as third word (cf. the | |||
[documentation for `expected_region_highlight`](docs/highlighters.md)). For example: | |||
```zsh | |||
ZSH_HIGHLIGHT_PATTERNS+=('rm -rf *' 'fg=white,bold,bg=red') | |||
BUFFER='rm -rf /' | |||
expected_region_highlight=( | |||
"1 8 fg=white,bold,bg=red" # rm -rf / | |||
) | |||
``` | |||
Memos and commas | |||
---------------- | |||
We append to `region_highlight` as follows: | |||
```zsh | |||
region_highlight+=("$start $end $spec, memo=zsh-syntax-highlighting") | |||
``` | |||
That comma is required to cause zsh 5.8 and older to ignore the memo without | |||
ignoring the `$spec`. It's a hack, but given that no further 5.8.x patch | |||
releases are planned, it's been deemed acceptable. See issue #418 and the | |||
cross-referenced issues. | |||
Miscellany | |||
---------- | |||
If you work on the driver (`zsh-syntax-highlighting.zsh`), you may find the following zstyle useful: | |||
```zsh | |||
zstyle ':completion:*:*:*:*:globbed-files' ignored-patterns {'*/',}zsh-syntax-highlighting.plugin.zsh | |||
``` | |||
IRC channel | |||
----------- | |||
We're on #zsh-syntax-highlighting on Libera.Chat. | |||
@ -1,131 +0,0 @@ | |||
How to install | |||
-------------- | |||
### Using packages | |||
* Arch Linux: [community/zsh-syntax-highlighting][arch-package] / [AUR/zsh-syntax-highlighting-git][AUR-package] | |||
* Debian: `zsh-syntax-highlighting` package [in `stretch`][debian-package] (or in [OBS repository][obs-repository]) | |||
* Fedora: [zsh-syntax-highlighting package][fedora-package-alt] in Fedora 24+ (or in [OBS repository][obs-repository]) | |||
* FreeBSD: `pkg install zsh-syntax-highlighting` (port name: [`shells/zsh-syntax-highlighting`][freebsd-port]) | |||
* Gentoo: [app-shells/zsh-syntax-highlighting][gentoo-repository] | |||
* Mac OS X / Homebrew: [brew install zsh-syntax-highlighting][brew-package] | |||
* NetBSD: `pkg_add zsh-syntax-highlighting` (port name: [`shells/zsh-syntax-highlighting`][netbsd-port]) | |||
* OpenBSD: `pkg_add zsh-syntax-highlighting` (port name: [`shells/zsh-syntax-highlighting`][openbsd-port]) | |||
* openSUSE / SLE: `zsh-syntax-highlighting` package in [OBS repository][obs-repository] | |||
* RHEL / CentOS / Scientific Linux: `zsh-syntax-highlighting` package in [OBS repository][obs-repository] | |||
* Ubuntu: `zsh-syntax-highlighting` package [in Xenial][ubuntu-package] (or in [OBS repository][obs-repository]) | |||
* Void Linux: `zsh-syntax-highlighting package` [in XBPS][void-package] | |||
[arch-package]: https://www.archlinux.org/packages/zsh-syntax-highlighting | |||
[AUR-package]: https://aur.archlinux.org/packages/zsh-syntax-highlighting-git | |||
[brew-package]: https://github.com/Homebrew/homebrew-core/blob/master/Formula/zsh-syntax-highlighting.rb | |||
[debian-package]: https://packages.debian.org/zsh-syntax-highlighting | |||
[fedora-package]: https://apps.fedoraproject.org/packages/zsh-syntax-highlighting | |||
[fedora-package-alt]: https://bodhi.fedoraproject.org/updates/?packages=zsh-syntax-highlighting | |||
[freebsd-port]: http://www.freshports.org/textproc/zsh-syntax-highlighting/ | |||
[gentoo-repository]: https://packages.gentoo.org/packages/app-shells/zsh-syntax-highlighting | |||
[netbsd-port]: http://cvsweb.netbsd.org/bsdweb.cgi/pkgsrc/shells/zsh-syntax-highlighting/ | |||
[obs-repository]: https://software.opensuse.org/download.html?project=shells%3Azsh-users%3Azsh-syntax-highlighting&package=zsh-syntax-highlighting | |||
[openbsd-port]: https://cvsweb.openbsd.org/ports/shells/zsh-syntax-highlighting/ | |||
[ubuntu-package]: https://launchpad.net/ubuntu/+source/zsh-syntax-highlighting | |||
[void-package]: https://github.com/void-linux/void-packages/tree/master/srcpkgs/zsh-syntax-highlighting | |||
See also [repology's cross-distro index](https://repology.org/metapackage/zsh-syntax-highlighting/versions) | |||
### In your ~/.zshrc | |||
Simply clone this repository and source the script: | |||
```zsh | |||
git clone https://github.com/zsh-users/zsh-syntax-highlighting.git | |||
echo "source ${(q-)PWD}/zsh-syntax-highlighting/zsh-syntax-highlighting.zsh" >> ${ZDOTDIR:-$HOME}/.zshrc | |||
``` | |||
Then, enable syntax highlighting in the current interactive shell: | |||
```zsh | |||
source ./zsh-syntax-highlighting/zsh-syntax-highlighting.zsh | |||
``` | |||
If `git` is not installed, download and extract a snapshot of the latest | |||
development tree from: | |||
``` | |||
https://github.com/zsh-users/zsh-syntax-highlighting/archive/master.tar.gz | |||
``` | |||
Note the `source` command must be **at the end** of `~/.zshrc`. | |||
### With a plugin manager | |||
Note that `zsh-syntax-highlighting` must be the last plugin sourced. | |||
The zsh-syntax-highlighting authors recommend manual installation over the use | |||
of a framework or plugin manager. | |||
This list is incomplete as there are too many | |||
[frameworks / plugin managers][framework-list] to list them all here. | |||
[framework-list]: https://github.com/unixorn/awesome-zsh-plugins#frameworks | |||
#### [Antigen](https://github.com/zsh-users/antigen) | |||
Add `antigen bundle zsh-users/zsh-syntax-highlighting` as the last bundle in | |||
your `.zshrc`. | |||
#### [Oh-my-zsh](https://github.com/robbyrussell/oh-my-zsh) | |||
1. Clone this repository in oh-my-zsh's plugins directory: | |||
```zsh | |||
git clone https://github.com/zsh-users/zsh-syntax-highlighting.git ${ZSH_CUSTOM:-~/.oh-my-zsh/custom}/plugins/zsh-syntax-highlighting | |||
``` | |||
2. Activate the plugin in `~/.zshrc`: | |||
```zsh | |||
plugins=( [plugins...] zsh-syntax-highlighting) | |||
``` | |||
3. Restart zsh (such as by opening a new instance of your terminal emulator). | |||
#### [Prezto](https://github.com/sorin-ionescu/prezto) | |||
Zsh-syntax-highlighting is included with Prezto. See the | |||
[Prezto documentation][prezto-docs] to enable and configure highlighters. | |||
[prezto-docs]: https://github.com/sorin-ionescu/prezto/tree/master/modules/syntax-highlighting | |||
#### [zgen](https://github.com/tarjoilija/zgen) | |||
Add `zgen load zsh-users/zsh-syntax-highlighting` to the end of your `.zshrc`. | |||
#### [zplug](https://github.com/zplug/zplug) | |||
Add `zplug "zsh-users/zsh-syntax-highlighting", defer:2` to your `.zshrc`. | |||
#### [zplugin](https://github.com/psprint/zplugin) | |||
Add `zplugin load zsh-users/zsh-syntax-highlighting` to the end of your | |||
`.zshrc`. | |||
### System-wide installation | |||
Any of the above methods is suitable for a single-user installation, | |||
which requires no special privileges. If, however, you desire to install | |||
zsh-syntax-highlighting system-wide, you may do so by running | |||
```zsh | |||
make install | |||
``` | |||
and directing your users to add | |||
```zsh | |||
source /usr/local/share/zsh-syntax-highlighting/zsh-syntax-highlighting.zsh | |||
``` | |||
to their `.zshrc`s. |
@ -1,64 +0,0 @@ | |||
NAME=zsh-syntax-highlighting | |||
INSTALL?=install -c | |||
PREFIX?=/usr/local | |||
SHARE_DIR?=$(DESTDIR)$(PREFIX)/share/$(NAME) | |||
DOC_DIR?=$(DESTDIR)$(PREFIX)/share/doc/$(NAME) | |||
ZSH?=zsh # zsh binary to run tests with | |||
all: | |||
cd docs && \ | |||
cp highlighters.md all.md && \ | |||
printf '\n\nIndividual highlighters documentation\n=====================================' >> all.md && \ | |||
for doc in highlighters/*.md; do printf '\n\n'; cat "$$doc"; done >> all.md | |||
install: all | |||
$(INSTALL) -d $(SHARE_DIR) | |||
$(INSTALL) -d $(DOC_DIR) | |||
cp .version zsh-syntax-highlighting.zsh $(SHARE_DIR) | |||
cp COPYING.md README.md changelog.md $(DOC_DIR) | |||
sed -e '1s/ .*//' -e '/^\[build-status-[a-z]*\]: /d' < README.md > $(DOC_DIR)/README.md | |||
if [ x"true" = x"`git rev-parse --is-inside-work-tree 2>/dev/null`" ]; then \ | |||
git rev-parse HEAD; \ | |||
else \ | |||
cat .revision-hash; \ | |||
fi > $(SHARE_DIR)/.revision-hash | |||
: | |||
# The [ -e ] check below is to because sh evaluates this with (the moral | |||
# equivalent of) NONOMATCH in effect, and highlighters/*.zsh has no matches. | |||
for dirname in highlighters highlighters/*/ ; do \ | |||
$(INSTALL) -d $(SHARE_DIR)/"$$dirname"; \ | |||
for fname in "$$dirname"/*.zsh ; do [ -e "$$fname" ] && cp "$$fname" $(SHARE_DIR)"/$$dirname"; done; \ | |||
done | |||
cp -R docs/* $(DOC_DIR) | |||
clean: | |||
rm -f docs/all.md | |||
test: | |||
@$(ZSH) -fc 'echo ZSH_PATCHLEVEL=$$ZSH_PATCHLEVEL' | |||
@result=0; \ | |||
for test in highlighters/*; do \ | |||
if [ -d $$test/test-data ]; then \ | |||
echo "Running test $${test##*/}"; \ | |||
env -i QUIET=$$QUIET $${TERM:+"TERM=$$TERM"} $(ZSH) -f tests/test-highlighting.zsh "$${test##*/}"; \ | |||
: $$(( result |= $$? )); \ | |||
fi \ | |||
done; \ | |||
exit $$result | |||
quiet-test: | |||
$(MAKE) test QUIET=y | |||
perf: | |||
@result=0; \ | |||
for test in highlighters/*; do \ | |||
if [ -d $$test/test-data ]; then \ | |||
echo "Running test $${test##*/}"; \ | |||
$(ZSH) -f tests/test-perfs.zsh "$${test##*/}"; \ | |||
: $$(( result |= $$? )); \ | |||
fi \ | |||
done; \ | |||
exit $$result | |||
.PHONY: all install clean test perf |
@ -1,97 +1,290 @@ | |||
zsh-syntax-highlighting [![Build Status][build-status-image]][build-status] | |||
======================= | |||
[](https://www.paypal.me/ZdharmaInitiative) | |||
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=D54B3S7C6HGME) | |||
[](https://www.patreon.com/psprint) | |||
<br/>New: You can request a feature when donating, even fancy or advanced ones get implemented this way. [There are | |||
reports](DONATIONS.md) about what is being done with the money received. | |||
**[Fish shell][fish]-like syntax highlighting for [Zsh][zsh].** | |||
# Fast Syntax Highlighting (F-Sy-H) | |||
*Requirements: zsh 4.3.11+.* | |||
Feature rich syntax highlighting for Zsh. | |||
[fish]: https://fishshell.com/ | |||
[zsh]: https://www.zsh.org/ | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/highlight-much.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
This package provides syntax highlighting for the shell zsh. It enables | |||
highlighting of commands whilst they are typed at a zsh prompt into an | |||
interactive terminal. This helps in reviewing commands before running | |||
them, particularly in catching syntax errors. | |||
### Table of Contents | |||
Some examples: | |||
- [News](#news) | |||
- [Installation](#installation) | |||
- [Features](#features) | |||
- [Performance](#performance) | |||
- [IRC Channel](#irc-channel) | |||
Before: [](images/before1.png) | |||
<br/> | |||
After: [](images/after1.png) | |||
### Other Contents | |||
- [License](https://github.com/zdharma/fast-syntax-highlighting/blob/master/LICENSE) | |||
- [Changelog](https://github.com/zdharma/fast-syntax-highlighting/blob/master/CHANGELOG.md) | |||
- [Theme Guide](https://github.com/zdharma/fast-syntax-highlighting/blob/master/THEME_GUIDE.md) | |||
- [Chroma Guide](https://github.com/zdharma/fast-syntax-highlighting/blob/master/CHROMA_GUIDE.adoc) | |||
Before: [](images/before2.png) | |||
<br/> | |||
After: [](images/after2.png) | |||
# News | |||
Before: [](images/before3.png) | |||
<br/> | |||
After: [](images/after3.png) | |||
* 15-06-2019 | |||
- A new architecture for defining the highlighting for **specific commands**: it now | |||
uses **abstract definitions** instead of **top-down, regular code**. The first effect | |||
is the highlighting for the `git` command it is now **maximally faithful**, it | |||
follows the `git` command almost completely. | |||
[Screencast](https://asciinema.org/a/253411) | |||
Before: [](images/before4-smaller.png) | |||
<br/> | |||
After: [](images/after4-smaller.png) | |||
# Installation | |||
### Manual | |||
Clone the Repository. | |||
How to install | |||
-------------- | |||
```zsh | |||
git clone https://github.com/zdharma/fast-syntax-highlighting ~/path/to/fsh | |||
``` | |||
See [INSTALL.md](INSTALL.md). | |||
And add the following to your `zshrc` file. | |||
```zsh | |||
source ~/path/to/fsh/fast-syntax-highlighting.plugin.zsh | |||
``` | |||
### Zinit | |||
FAQ | |||
--- | |||
Add the following to your `zshrc` file. | |||
### Why must `zsh-syntax-highlighting.zsh` be sourced at the end of the `.zshrc` file? | |||
```zsh | |||
zinit light zdharma/fast-syntax-highlighting | |||
``` | |||
zsh-syntax-highlighting works by hooking into the Zsh Line Editor (ZLE) and | |||
computing syntax highlighting for the command-line buffer as it stands at the | |||
time z-sy-h's hook is invoked. | |||
Here's an example of how to load the plugin together with a few other popular | |||
ones with the use of | |||
[Turbo](https://zdharma.org/zinit/wiki/INTRODUCTION/#turbo_mode_zsh_62_53), | |||
i.e.: speeding up the Zsh startup by loading the plugin right after the first | |||
prompt, in background: | |||
In zsh 5.2 and older, | |||
`zsh-syntax-highlighting.zsh` hooks into ZLE by wrapping ZLE widgets. It must | |||
be sourced after all custom widgets have been created (i.e., after all `zle -N` | |||
calls and after running `compinit`) in order to be able to wrap all of them. | |||
Widgets created after z-sy-h is sourced will work, but will not update the | |||
syntax highlighting. | |||
```zsh | |||
zinit wait lucid for \ | |||
atinit"ZINIT[COMPINIT_OPTS]=-C; zicompinit; zicdreplay" \ | |||
zdharma/fast-syntax-highlighting \ | |||
blockf \ | |||
zsh-users/zsh-completions \ | |||
atload"!_zsh_autosuggest_start" \ | |||
zsh-users/zsh-autosuggestions | |||
``` | |||
In zsh newer than 5.8 (not including 5.8 itself), | |||
zsh-syntax-highlighting uses the `add-zle-hook-widget` facility to install | |||
a `zle-line-pre-redraw` hook. Hooks are run in order of registration, | |||
therefore, z-sy-h must be sourced (and register its hook) after anything else | |||
that adds hooks that modify the command-line buffer. | |||
### Antigen | |||
### Does syntax highlighting work during incremental history search? | |||
Add the following to your `zshrc` file. | |||
Highlighting the command line during an incremental history search (by default bound to | |||
to <kbd>Ctrl+R</kbd> in zsh's emacs keymap) requires zsh 5.4 or newer. | |||
```zsh | |||
antigen bundle zdharma/fast-syntax-highlighting | |||
``` | |||
Under zsh versions older than 5.4, the zsh-default [underlining][zshzle-Character-Highlighting] | |||
of the matched portion of the buffer remains available, but zsh-syntax-highlighting's | |||
additional highlighting is unavailable. (Those versions of zsh do not provide | |||
enough information to allow computing the highlighting correctly.) | |||
### Zgen | |||
See issues [#288][i288] and [#415][i415] for details. | |||
Add the following to your `.zshrc` file in the same place you're doing | |||
your other `zgen load` calls in. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting | |||
[i288]: https://github.com/zsh-users/zsh-syntax-highlighting/pull/288 | |||
[i415]: https://github.com/zsh-users/zsh-syntax-highlighting/pull/415 | |||
```zsh | |||
zgen load zdharma/fast-syntax-highlighting | |||
``` | |||
### How are new releases announced? | |||
There is currently no "push" announcements channel. However, the following | |||
alternatives exist: | |||
### Oh-My-Zsh | |||
- GitHub's RSS feed of releases: https://github.com/zsh-users/zsh-syntax-highlighting/releases.atom | |||
- An anitya entry: https://release-monitoring.org/project/7552/ | |||
Clone the Repository. | |||
```zsh | |||
git clone https://github.com/zdharma/fast-syntax-highlighting.git \ | |||
${ZSH_CUSTOM:-$HOME/.oh-my-zsh/custom}/plugins/fast-syntax-highlighting | |||
``` | |||
How to tweak | |||
------------ | |||
And add `fast-syntax-highlighting` to your plugin list. | |||
Syntax highlighting is done by pluggable highlighter scripts. See the | |||
[documentation on highlighters](docs/highlighters.md) for details and | |||
configuration settings. | |||
# Features | |||
[build-status]: https://github.com/zsh-users/zsh-syntax-highlighting/actions | |||
[build-status-image]: https://github.com/zsh-users/zsh-syntax-highlighting/workflows/Tests/badge.svg | |||
### Themes | |||
Switch themes via `fast-theme {theme-name}`. | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/theme.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
Run `fast-theme -t {theme-name}` option to obtain the snippet above. | |||
Run `fast-theme -l` to list available themes. | |||
### Variables | |||
Comparing to the project `zsh-users/zsh-syntax-highlighting` (the upper line): | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/parameter.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/in_string.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Brackets | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/brackets.gif" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Conditions | |||
Comparing to the project `zsh-users/zsh-syntax-highlighting` (the upper line): | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/cplx_cond.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Strings | |||
Exact highlighting that recognizes quotings. | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/ideal-string.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### here-strings | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/herestring.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### `exec` descriptor-variables | |||
Comparing to the project `zsh-users/zsh-syntax-highlighting` (the upper line): | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/execfd_cmp.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### for-loops and alternate syntax (brace `{`/`}` blocks) | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/for-loop-cmp.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Function definitions | |||
Comparing to the project `zsh-users/zsh-syntax-highlighting` (the upper 2 lines): | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/function.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Recursive `eval` and `$( )` highlighting | |||
Comparing to the project `zsh-users/zsh-syntax-highlighting` (the upper line): | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/eval_cmp.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Chroma functions | |||
Highlighting that is specific for a given command. | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/git_chroma.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
The [chromas](https://github.com/zdharma/fast-syntax-highlighting/tree/master/chroma) | |||
that are enabled by default can be found | |||
[here](https://github.com/zdharma/fast-syntax-highlighting/blob/master/fast-highlight#L166). | |||
### Math-mode highlighting | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/math.gif" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
### Zcalc highlighting | |||
<div style="width:100%;background-color:black;border:3px solid black;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<img | |||
src="https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/images/zcalc.png" | |||
alt="image could not be loaded" | |||
style="color:red;background-color:black;font-weight:bold" | |||
/> | |||
</div> | |||
# Performance | |||
Performance differences can be observed in this Asciinema recording, where a `10 kB` function is being edited. | |||
<div style="width:100%;background-color:#121314;border:3px solid #121314;border-radius:6px;margin:5px 0;padding:2px 5px"> | |||
<a href="https://asciinema.org/a/112367"> | |||
<img src="https://asciinema.org/a/112367.png" alt="asciicast"> | |||
</a> | |||
</div> | |||
## IRC Channel | |||
Channel `#zinit@freenode` is a support place for all author's projects. Connect to: | |||
[chat.freenode.net:6697](ircs://chat.freenode.net:6697/%23zinit) (SSL) or [chat.freenode.net:6667](irc://chat.freenode.net:6667/%23zinit) | |||
and join #zinit. | |||
Following is a quick access via Webchat [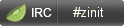](https://kiwiirc.com/client/chat.freenode.net:+6697/#zinit) |
@ -0,0 +1,76 @@ | |||
# Theme Guide for F-Sy-H | |||
`fast-theme` tool is used to select a theme. There are 6 shipped themes, they can be listed with `fast-theme -l`. | |||
Themes are basic [INI files](https://github.com/zdharma/fast-syntax-highlighting/tree/master/themes) where each | |||
key is a *style*. | |||
Besides shipped themes, user can point this tool to any other theme, by simple `fast-theme ~/mytheme.ini`. To | |||
obtain template to work on when creating own theme, issue `fast-theme --copy-shipped-theme {theme-name}`. | |||
To alter just a few styles and not create a whole new theme, use **overlay**. What is overlay? It is in the same | |||
format as full theme, but can have only a few styles defined, and these styles will overwrite styles in main-theme. | |||
Example overlay file: | |||
```ini | |||
; overlay.ini | |||
[base] | |||
commandseparator = yellow,bold | |||
comment = 17 | |||
[command-point] | |||
function = green | |||
command = 180 | |||
``` | |||
File name `overlay.ini` is treated specially. | |||
When specifing path, following short-hands can be used: | |||
``` | |||
XDG: = ~/.config/fsh (respects $XDG_CONFIG_HOME env var) | |||
LOCAL: = /usr/local/share/fsh/ | |||
HOME: = ~/.fsh/ | |||
OPT: = /opt/local/share/fsh/ | |||
``` | |||
So for example, issue `fast-theme XDG:overlay` to load `~/.config/fsh/overlay.ini` as overlay. The `.ini` | |||
extension is optional. | |||
## Secondary Theme | |||
Each theme has key `secondary`, e.g. for theme `free`: | |||
```ini | |||
; free.ini | |||
[base] | |||
default = none | |||
unknown-token = red,bold | |||
; ... | |||
; ... | |||
; ... | |||
secondary = zdharma | |||
``` | |||
Secondary theme (`zdharma` in the example) will be used for highlighting of argument for `eval` | |||
and of `$( ... )` interior (i.e. of interior of command substitution). Basically, recursive | |||
highlighting uses alternate theme to make the highlighted code distinct: | |||
 | |||
In the above screen-shot the interior of `$( ... )` uses different colors than the rest of the | |||
code. Example for `eval`: | |||
 | |||
First line doesn't use recursive highlighting, highlights `eval` argument as regular string. | |||
Second line switches theme to `zdharma` and does full recursive highlighting of eval argument. | |||
## Custom Working Directory | |||
Set `$FAST_WORK_DIR` before loading the plugin to have e.g. processed theme files (ready to | |||
load, in Zsh format, not INI) kept under specified location. This is handy if e.g. you install | |||
Fast-Syntax-Highlighting system-wide (e.g. from AUR on ArchLinux) and want to have per-user | |||
theme setup. | |||
You can use "~" in the path, e.g. `FAST_WORK_DIR=~/.fsh` and also the `XDG:`, `LOCAL:`, `OPT:`, | |||
etc. short-hands, so e.g. `FAST_WORK_DIR=XDG` or `FAST_WORK_DIR=XDG:` is allowed (in this case | |||
it will be changed to `$HOME/.config/fsh` by default by F-Sy-H loader). |
@ -0,0 +1,39 @@ | |||
#compdef fast-theme | |||
# | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
# | |||
# Completion for theme-switching function, fast-theme, | |||
# part of zdharma/fast-syntax-highlighting. | |||
# | |||
integer ret=1 | |||
local -a arguments | |||
arguments=( | |||
{-h,--help}'[display help text]' | |||
{-l,--list}'[list available themes]' | |||
{-r,--reset}'[unset any theme (revert to default highlighting)]' | |||
{-R,--ov-reset}'[unset overlay, use styles only from main-theme (requires restart)]' | |||
{-q,--quiet}'[no default messages]' | |||
{-s,--show}'[get and display the theme currently being set]' | |||
{-v,--verbose}'[more messages during operation]' | |||
{-t,--test}'[test theme after setting it (show example code)]' | |||
{-p,--palette}'[just print all 256 colors and exit (useful when creating a theme)]' | |||
{-w,--workdir}'[cd into $FAST_WORK_DIR (if not set, then into the plugin directory)]' | |||
) | |||
typeset -a themes | |||
themes=( "$FAST_WORK_DIR"/themes/*.ini(:t:r) ) | |||
if [[ -d ${XDG_CONFIG_HOME:-$HOME/.config}/fsh ]] { | |||
typeset -a themes2 | |||
themes2=( "${XDG_CONFIG_HOME:-$HOME/.config}"/fsh/*.ini(:t:r) ) | |||
themes+=( XDG:${^themes2[@]} ) | |||
} | |||
_wanted themes expl "Themes" \ | |||
compadd "$@" -a - themes && ret=0 | |||
_arguments -s $arguments && ret=0 | |||
return $ret |
@ -1,884 +0,0 @@ | |||
# Changes in HEAD | |||
## Changes fixed as part of the switch to zle-line-pre-redraw | |||
The changes in this section were fixed by switching to a `zle-line-pre-redraw`-based | |||
implementation. | |||
Note: The new implementation will only be used on future zsh releases, | |||
numbered 5.8.0.3 and newer, due to interoperability issues with other plugins | |||
(issues #418 and #579). The underlying zsh feature has been available since | |||
zsh 5.2. | |||
Whilst under development, the new implementation was known as the | |||
"feature/redrawhook" topic branch. | |||
- Fixed: Highlighting not triggered after popping a buffer from the buffer stack | |||
(using the `push-line` widget, default binding: `M-q`) | |||
[#40] | |||
- Fixed: Invoking completion when there were no matches removed highlighting | |||
[#90, #470] | |||
- Fixed: Two successive deletes followed by a yank only yanked the latest | |||
delete, rather than both of them | |||
[#150, #151, #160; cf. #183] | |||
- Presumed fixed: Completing `$(xsel)` results in an error message from `xsel`, | |||
with pre-2017 versions of `xsel`. (For 2017 vintage and newer, see the issue | |||
for details.) | |||
[#154] | |||
- Fixed: When the standard `bracketed-paste-magic` widget is in use, pastes were slow | |||
[#295] | |||
- Fixed: No way to prevent a widget from being wrapped | |||
[#324] | |||
- Fixed: No highlighting while cycling menu completion | |||
[#375] | |||
- Fixed: Does not coexist with the `IGNORE_EOF` option | |||
[#377] | |||
- Fixed: The `undefined-key` widget was wrapped | |||
[#421] | |||
- Fixed: Does not coexist with the standard `surround` family of widgets | |||
[#520] | |||
- Fixed: First completed filename doesn't get `path` highlighting | |||
[#632] | |||
## Other changes | |||
- Add issue #712 to the previous release's changelog (hereinafter). | |||
# Changes in 0.8.0-alpha1-pre-redrawhook | |||
## Notice about an improbable-but-not-impossible forward incompatibility | |||
Everyone can probably skip this section. | |||
The `master` branch of zsh-syntax-highlighting uses a zsh feature that has not | |||
yet appeared in a zsh release: the `memo=` feature, added to zsh in commit | |||
zsh-5.8-172-gdd6e702ee (after zsh 5.8, before zsh 5.9). In the unlikely event | |||
that this zsh feature should change in an incompatible way before the next | |||
stable zsh release, set `zsh_highlight__memo_feature=0` in your .zshrc files to | |||
disable use of the new feature. | |||
z-sy-h dogfoods the new, unreleased zsh feature because that feature was | |||
added to zsh at z-sy-h's initiative. The new feature is used in the fix | |||
to issue #418. | |||
## Incompatible changes: | |||
- An unsuccessful completion (a <kbd>⮀ Tab</kbd> press that doesn't change the | |||
command line) no longer causes highlighting to be lost. Visual feedback can | |||
alternatively be achieved by setting the `format` zstyle under the `warnings` | |||
tag, for example, | |||
zstyle ':completion:*:warnings' format '%F{red}No matches%f' | |||
Refer to the [description of the `format` style in `zshcompsys(1)`] | |||
[zshcompsys-Standard-Styles-format]. | |||
(#90, part of #245 (feature/redrawhook)) | |||
[zshcompsys-Standard-Styles]: http://zsh.sourceforge.net/Doc/Release/Completion-System.html#Standard-Styles | |||
[zshcompsys-Standard-Styles-format]: http://zsh.sourceforge.net/Doc/Release/Completion-System.html#index-format_002c-completion-style | |||
## Other changes: | |||
- Document `$ZSH_HIGHLIGHT_MAXLENGTH`. | |||
[#698] | |||
- Optimize highlighting unquoted words (words that are not in single quotes, double quotes, backticks, or dollar-single-quotes) | |||
[#730] | |||
- Redirection operators (e.g., `<` and `>`) are now highlighted by default | |||
[#646] | |||
- Propertly terminate `noglob` scope in try/always blocks | |||
[#577] | |||
- Don't error out when `KSH_ARRAYS` is set in the calling scope | |||
[#622, #689] | |||
- Literal semicolons in array assignments (`foo=( bar ; baz )`) are now | |||
highlighted as errors. | |||
[3ca93f864fb6] | |||
- Command separators in array assignments (`foo=( bar | baz )`) are now | |||
highlighted as errors. | |||
[#651, 81267ca3130c] | |||
- Support parameter elision in command position (e.g., `$foo ls` where `$foo` is unset or empty) | |||
[#667] | |||
- Don't consider the filename in `sudo -e /path/to/file` to be a command position | |||
[#678] | |||
- Don't look up absolute directory names in $cdpath | |||
[2cc2583f8f12, part of #669] | |||
- Fix `exec 2>&1;` being highlighted as an error. | |||
[#676] | |||
- Fix `: $(<*)` being highlighted as globbing. | |||
[#582] | |||
- Fix `cat < *` being highlighting as globbing when the `MULTIOS` option is unset. | |||
[#583] | |||
- Fix `echo >&2` highlighting the `2` as a filename if a file by that name happened to exist | |||
[#694, part of #645] | |||
- Fix `echo >&-` highlighting the `-` as a filename if a file by that name happened to exist | |||
[part of #645] | |||
- Fix `echo >&p` highlighting the `p` as a filename if a file by that name happened to exist | |||
[part of #645] | |||
- Fix wrong highlighting of unquoted parameter expansions under zsh 5.2 and older | |||
[e165f18c758e] | |||
- Highlight global aliases | |||
[#700] | |||
- Highlight `: =nosuchcommand' as an error (when the `EQUALS` option hasn't been unset). | |||
[#430] | |||
- Highlight reserved word after assignments as errors (e.g., `foo=bar (ls;)`) | |||
[#461] | |||
- Correctly highlight `[[ foo && bar || baz ]]`. | |||
- Highlight non-executable files in command position correctly (e.g., `% /etc/passwd`) | |||
[#202, #669] | |||
- Highlight directories in command position correctly, including `AUTO_CD` support | |||
[#669] | |||
- Recognize `env` as a precommand (e.g., `env FOO=bar ls`) | |||
- Recognize `strace` as a precommand | |||
- Fix an error message on stderr before every prompt when the `WARN_NESTED_VAR` zsh option is set: | |||
`_zsh_highlight_main__precmd_hook:1: array parameter _zsh_highlight_main__command_type_cache set in enclosing scope in function _zsh_highlight_main__precmd_hook` | |||
[#727, #731, #732, #733] | |||
- Fix highlighting of alias whose definitions use a simple command terminator | |||
(such as `;`, `|`, `&&`) before a newline | |||
[#677; had regressed in 0.7.0] | |||
- Highlight arithmetic expansions (e.g., `$(( 42 ))`) | |||
[#607 #649 #704] | |||
- Highlight the parentheses of array assignments as reserved words (`foo=( bar )`). | |||
The `assign` style remains supported and has precedence. | |||
[#585] | |||
- Fix interoperability issue with other plugins that use highlighting. The fix | |||
requires zsh 5.8.0.3 or newer. (zsh 5.8.0.2-dev from the `master` branch, | |||
revision zsh-5.8-172-gdd6e702ee or newer is also fine.) | |||
[#418, https://github.com/okapia/zsh-viexchange/issues/1] | |||
- Improve performance of the `brackets` highlighter. | |||
- Fix highlighting of pre-command redirections (e.g., the `$fn` in `<$fn cat`) | |||
[#712] | |||
# Changes in version 0.7.1 | |||
- Remove out-of-date information from the 0.7.0 changelog. | |||
# Changes in version 0.7.0 | |||
This is a stable bugfix and feature release. Major new features and changes include: | |||
- Add `ZSH_HIGHLIGHT_DIRS_BLACKLIST` to disable "path" and "path prefix" | |||
highlighting for specific directories | |||
[#379] | |||
- Add the "regexp" highlighter, modelled after the pattern highlighter | |||
[4e6f60063f1c] | |||
- When a word uses globbing, only the globbing metacharacters will be highlighted as globbing: | |||
in `: foo*bar`, only the `*` will be blue. | |||
[e48af357532c] | |||
- Highlight pasted quotes (e.g., `: foo"bar"`) | |||
[dc1b2f6fa4bb] | |||
- Highlight command substitutions (`` : `ls` ``, `: $(ls)`) | |||
[c0e64fe13178 and parents, e86f75a840e7, et al] | |||
- Highlight process substitutions (`: >(nl)`, `: <(pwd)`, `: =(git diff)`) | |||
[c0e64fe13178 and parents, e86f75a840e7, et al] | |||
- Highlight command substitutions inside double quotes (``: "`foo`"``) | |||
[f16e858f0c83] | |||
- Highlight many precommands (e.g., `nice`, `stdbuf`, `eatmydata`; | |||
see `$precommand_options` in the source) | |||
- Highlight numeric globs (e.g., `echo /lib<->`) | |||
- Assorted improvements to aliases highlighting | |||
(e.g., | |||
`alias sudo_u='sudo -u'; sudo_u jrandom ls`, | |||
`alias x=y y=z z=nosuchcommand; x`, | |||
`alias ls='ls -l'; \ls`) | |||
[f3410c5862fc, 57386f30aec8, #544, and many others] | |||
- Highlight some more syntax errors | |||
[dea05e44e671, 298ef6a2fa30] | |||
- New styles: named file descriptors, `RC_QUOTES`, and unclosed quotes (e.g., `echo "foo<CURSOR>`) | |||
[38c794a978cd, 25ae1c01216c, 967335dfc5fd] | |||
- The 'brackets' highlighting no longer treats quotes specially. | |||
[ecdda36ef56f] | |||
Selected bugfixes include: | |||
- Highlight `sudo` correctly when it's not installed | |||
[26a82113b08b] | |||
- Handle some non-default options being set in zshrc | |||
[b07ada1255b7, a2a899b41b8, 972ad197c13d, b3f66fc8748f] | |||
- Fix off-by-one highlighting in vi "visual" mode (vicmd keymap) | |||
[be3882aeb054] | |||
- The 'yank-pop' widget is not wrapped | |||
[#183] | |||
Known issues include: | |||
- A multiline alias that uses a simple command terminator (such as `;`, `|`, `&&`) | |||
before a newline will incorrectly be highlighted as an error. See issue #677 | |||
for examples and workarounds. | |||
[#677] | |||
[UPDATE: Fixed in 0.8.0] | |||
# Changes in version 0.6.0 | |||
This is a stable release, featuring bugfixes and minor improvements. | |||
## Performance improvements: | |||
(none) | |||
## Added highlighting of: | |||
- The `isearch` and `suffix` [`$zle_highlight` settings][zshzle-Character-Highlighting]. | |||
(79e4d3d12405, 15db71abd0cc, b56ee542d619; requires zsh 5.3 for `$ISEARCHMATCH_ACTIVE` / `$SUFFIX_ACTIVE` support) | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting | |||
- Possible history expansions in double-quoted strings. | |||
(76ea9e1df316) | |||
- Mismatched `if`/`then`/`elif`/`else`/`fi`. | |||
(73cb83270262) | |||
## Fixed highlighting of: | |||
- A comment line followed by a non-comment line. | |||
(#385, 9396ad5c5f9c) | |||
- An unquoted `$*` (expands to the positional parameters). | |||
(237f89ad629f) | |||
- history-incremental-pattern-search-backward under zsh 5.3.1. | |||
(#407, #415, 462779629a0c) | |||
## API changes (for highlighter authors): | |||
(none) | |||
## Developer-visible changes: | |||
- tests: Set the `ALIAS_FUNC_DEF` option for zsh 5.4 compatibility. | |||
(9523d6d49cb3) | |||
## Other changes: | |||
- docs: Added before/after screenshots. | |||
(cd9ec14a65ec..b7e277106b49) | |||
- docs: Link Fedora package. | |||
(3d74aa47e4a7, 5feed23962df) | |||
- docs: Link FreeBSD port. | |||
(626c034c68d7) | |||
- docs: Link OpenSUSE Build Service packages | |||
(#419, dea1fedc7358) | |||
- Prevent user-defined aliases from taking effect in z-sy-h's own code. | |||
(#390, 2dce602727d7, 8d5afe47f774; and #392, #395, b8fa1b9dc954) | |||
- docs: Update zplug installation instructions. | |||
(#399, 4f49c4a35f17) | |||
- Improve "unhandled ZLE widget 'foo'" error message. | |||
(#409, be083d7f3710) | |||
- Fix printing of "failed loading highlighters" error message. | |||
(#426, ad522a091429) | |||
# Changes in version 0.5.0 | |||
## Performance improvements: | |||
We thank Sebastian Gniazdowski and "m0viefreak" for significant contributions | |||
in this area. | |||
- Optimize string operations in the `main` (default) highlighter. | |||
(#372/3cb58fd7d7b9, 02229ebd6328, ef4bfe5bcc14, #372/c6b6513ac0d6, #374/15461e7d21c3) | |||
- Command word highlighting: Use the `zsh/parameter` module to avoid forks. | |||
Memoize (cache) the results. | |||
(#298, 3ce01076b521, 2f18ba64e397, 12b879caf7a6; #320, 3b67e656bff5) | |||
- Avoid forks in the driver and in the `root` highlighter. | |||
(b9112aec798a, 38c8fbea2dd2) | |||
## Added highlighting of: | |||
- `pkexec` (a precommand). | |||
(#248, 4f3910cbbaa5) | |||
- Aliases that cannot be defined normally nor invoked normally (highlighted as an error). | |||
(#263 (in part), 28932316cca6) | |||
- Path separators (`/`) — the default behaviour remains to highlight path separators | |||
and path components the same way. | |||
(#136, #260, 6cd39e7c70d3, 9a934d291e7c, f3d3aaa00cc4) | |||
- Assignments to individual positional arguments (`42=foo` to assign to `$42`). | |||
(f4036a09cee3) | |||
- Linewise region (the `visual-line-mode` widget, bound to `V` in zsh's `vi` keymap). | |||
(#267, a7a7f8b42280, ee07588cfd9b) | |||
- Command-lines recalled by `isearch` mode; requires zsh≥5.3. | |||
(#261 (in part); #257; 4ad311ec0a68) | |||
- Command-lines whilst the `IGNORE_BRACES` or `IGNORE_CLOSE_BRACES` option is in effect. | |||
(a8a6384356af, 02807f1826a5) | |||
- Mismatched parentheses and braces (in the `main` highlighter). | |||
(51b9d79c3bb6, 2fabf7ca64b7, a4196eda5e6f, and others) | |||
- Mismatched `do`/`done` keywords. | |||
(b2733a64da93) | |||
- Mismatched `foreach`/`end` keywords. | |||
(#96, 2bb8f0703d8f) | |||
- In Bourne-style function definitions, when the `MULTI_FUNC_DEF` option is set | |||
(which is the default), highlight the first word in the function body as | |||
a command word: `f() { g "$@" }`. | |||
(6f91850a01e1) | |||
- `always` blocks. | |||
(#335, e5782e4ddfb6) | |||
- Command substitutions inside double quotes, `"$(echo foo)"`. | |||
(#139 (in part), c3913e0d8ead) | |||
- Non-alphabetic parameters inside double quotes (`"$$"`, `"$#"`, `"$*"`, `"$@"`, `"$?"`, `"$-"`). | |||
(4afe670f7a1b, 44ef6e38e5a7) | |||
- Command words from future versions of zsh (forward compatibly). | |||
This also adds an `arg0` style that all other command word styles fall back to. | |||
(b4537a972eed, bccc3dc26943) | |||
- Escaped history expansions inside double quotes: `: "\!"` | |||
(28d7056a7a06, et seq) | |||
## Fixed highlighting of: | |||
- Command separator tokens in syntactically-invalid positions. | |||
(09c4114eb980) | |||
- Redirections with a file descriptor number at command word. | |||
(#238 (in part), 73ee7c1f6c4a) | |||
- The `select` prompt, `$PS3`. | |||
(#268, 451665cb2a8b) | |||
- Values of variables in `vared`. | |||
(e500ca246286) | |||
- `!` as an argument (neither a history expansion nor a reserved word). | |||
(4c23a2fd1b90) | |||
- "division by zero" error under the `brackets` highlighter when `$ZSH_HIGHLIGHT_STYLES` is empty. | |||
(f73f3d53d3a6) | |||
- Process substitutions, `<(pwd)` and `>(wc -l)`. | |||
(#302, 6889ff6bd2ad, bfabffbf975c, fc9c892a3f15) | |||
- The non-`SHORT_LOOPS` form of `repeat` loops: `repeat 42; do true; done`. | |||
(#290, 4832f18c50a5, ef68f50c048f, 6362c757b6f7) | |||
- Broken symlinks (are now highlighted as files). | |||
(#342, 95f7206a9373, 53083da8215e) | |||
- Lines accepted from `isearch` mode. | |||
(#284; #257, #259, #288; 5bae6219008b, a8fe22d42251) | |||
- Work around upstream bug that triggered when the command word was a relative | |||
path, that when interpreted relative to a $PATH directory denoted a command; | |||
the effect of that upstream bug was that the relative path was cached as | |||
a "valid external command name". | |||
(#354, #355, 51614ca2c994, fdaeec45146b, 7d38d07255e4; | |||
upstream fix slated to be released in 5.3 (workers/39104)) | |||
- After accepting a line with the cursor on a bracket, the matching bracket | |||
of the bracket under the cursor no longer remains highlighted (with the | |||
`brackets` highlighter). | |||
(4c4baede519a) | |||
- The first word on a new line within an array assignment or initialization is no | |||
longer considered a command position. | |||
(8bf423d16d46) | |||
- Subshells that end at command position, `(A=42)`, `(true;)`. | |||
(#231, 7fb6f9979121; #344, 4fc35362ee5a) | |||
- Command word after array assignment, `a=(lorem ipsum) pwd`. | |||
(#330, 7fb6f9979121) | |||
## API changes (for highlighter authors): | |||
- New interface `_zsh_highlight_add_highlight`. | |||
(341a3ae1f015, c346f6eb6fb6) | |||
- tests: Specify the style key, not its value, in test expectations. | |||
(a830613467af, fd061b5730bf, eaa4335c3441, among others) | |||
- Module author documentation improvements. | |||
(#306 (in part), 217669270418, 0ff354b44b6e, 80148f6c8402, 364f206a547f, and others) | |||
- The driver no longer defines a `_zsh_highlight_${highlighter}_highlighter_cache` | |||
variable, which is in the highlighters' namespace. | |||
(3e59ab41b6b8, 80148f6c8402, f91a7b885e7d) | |||
- Rename highlighter entry points. The old names remain supported for | |||
backwards compatibility. | |||
(a3d5dfcbdae9, c793e0dceab1) | |||
- tests: Add the "NONE" expectation. | |||
(4da9889d1545, 13018f3dd735, d37c55c788cd) | |||
- tests: consider a test that writes to stderr to have failed. | |||
(#291, 1082067f9315) | |||
## Developer-visible changes: | |||
- Add `make quiet-test`. | |||
(9b64ad750f35) | |||
- test harness: Better quote replaceables in error messages. | |||
(30d8f92df225) | |||
- test harness: Fix exit code for XPASS. | |||
(bb8d325c0cbd) | |||
- Create [HACKING.md](HACKING.md). | |||
(cef49752fd0e) | |||
- tests: Emit a description for PASS test points. | |||
(6aa57d60aa64, f0bae44b76dd) | |||
- tests: Create a script that generates a test file. | |||
(8013dc3b8db6, et seq; `tests/generate.zsh`) | |||
## Other changes: | |||
- Under zsh≤5.2, widgets whose names start with a `_` are no longer excluded | |||
from highlighting. | |||
(ed33d2cb1388; reverts part of 186d80054a40 which was for #65) | |||
- Under zsh≤5.2, widgets implemented by a function named after the widget are | |||
no longer excluded from highlighting. | |||
(487b122c480d; reverts part of 776453cb5b69) | |||
- Under zsh≤5.2, shell-unsafe widget names can now be wrapped. | |||
(#278, 6a634fac9fb9, et seq) | |||
- Correct some test expectations. | |||
(78290e043bc5) | |||
- `zsh-syntax-highlighting.plugin.zsh`: Convert from symlink to plain file | |||
for msys2 compatibility. | |||
(#292, d4f8edc9f3ad) | |||
- Document installation under some plugin managers. | |||
(e635f766bef9, 9cab566f539b) | |||
- Don't leak the `PATH_DIRS` option. | |||
(7b82b88a7166) | |||
- Don't require the `FUNCTION_ARGZERO` option to be set. | |||
(#338, 750aebc553f2) | |||
- Under zsh≤5.2, support binding incomplete/nonexistent widgets. | |||
(9e569bb0fe04, part of #288) | |||
- Make the driver reentrant, fixing possibility of infinite recursion | |||
under zsh≤5.2 under interaction with theoretical third-party code. | |||
(#305, d711563fe1bf, 295d62ec888d, f3242cbd6aba) | |||
- Fix warnings when `WARN_CREATE_GLOBAL` is set prior to sourcing zsh-syntax-highlighting. | |||
(z-sy-h already sets `WARN_CREATE_GLOBAL` internally.) | |||
(da60234fb236) | |||
- Warn only once, rather than once per keypress, when a highlighter is unavailable. | |||
(0a9b347483ae) | |||
# Changes in version 0.4.1 | |||
## Fixes: | |||
- Arguments to widgets were not properly dash-escaped. Only matters for widgets | |||
that take arguments (i.e., that are invoked as `zle ${widget} -- ${args}`). | |||
(282c7134e8ac, reverts c808d2187a73) | |||
# Changes in version 0.4.0 | |||
## Added highlighting of: | |||
- incomplete sudo commands | |||
(a3047a912100, 2f05620b19ae) | |||
```zsh | |||
sudo; | |||
sudo -u; | |||
``` | |||
- command words following reserved words | |||
(#207, #222, b397b12ac139 et seq, 6fbd2aa9579b et seq, 8b4adbd991b0) | |||
```zsh | |||
if ls; then ls; else ls; fi | |||
repeat 10 do ls; done | |||
``` | |||
(The `ls` are now highlighted as a command.) | |||
- comments (when `INTERACTIVE_COMMENTS` is set) | |||
(#163, #167, 693de99a9030) | |||
```zsh | |||
echo Hello # comment | |||
``` | |||
- closing brackets of arithmetic expansion, subshells, and blocks | |||
(#226, a59f442d2d34, et seq) | |||
```zsh | |||
(( foo )) | |||
( foo ) | |||
{ foo } | |||
``` | |||
- command names enabled by the `PATH_DIRS` option | |||
(#228, 96ee5116b182) | |||
```zsh | |||
# When ~/bin/foo/bar exists, is executable, ~/bin is in $PATH, | |||
# and 'setopt PATH_DIRS' is in effect | |||
foo/bar | |||
``` | |||
- parameter expansions with braces inside double quotes | |||
(#186, 6e3720f39d84) | |||
```zsh | |||
echo "${foo}" | |||
``` | |||
- parameter expansions in command word | |||
(#101, 4fcfb15913a2) | |||
```zsh | |||
x=/bin/ls | |||
$x -l | |||
``` | |||
- the command separators '\|&', '&!', '&\|' | |||
```zsh | |||
view file.pdf &! ls | |||
``` | |||
## Fixed highlighting of: | |||
- precommand modifiers at non-command-word position | |||
(#209, 2c9f8c8c95fa) | |||
```zsh | |||
ls command foo | |||
``` | |||
- sudo commands with infix redirections | |||
(#221, be006aded590, 86e924970911) | |||
```zsh | |||
sudo -u >/tmp/foo.out user ls | |||
``` | |||
- subshells; anonymous functions | |||
(#166, #194, 0d1bfbcbfa67, 9e178f9f3948) | |||
```zsh | |||
(true) | |||
() { true } | |||
``` | |||
- parameter assignment statements with no command | |||
(#205, 01d7eeb3c713) | |||
```zsh | |||
A=1; | |||
``` | |||
(The semicolon used to be highlighted as a mistake) | |||
- cursor highlighter: Remove the cursor highlighting when accepting a line. | |||
(#109, 4f0c293fdef0) | |||
## Removed features: | |||
- Removed highlighting of approximate paths (`path_approx`). | |||
(#187, 98aee7f8b9a3) | |||
## Other changes: | |||
- main highlighter refactored to use states rather than booleans. | |||
(2080a441ac49, et seq) | |||
- Fix initialization when sourcing `zsh-syntax-highlighting.zsh` via a symlink | |||
(083c47b00707) | |||
- docs: Add screenshot. | |||
(57624bb9f64b) | |||
- widgets wrapping: Don't add '--' when invoking widgets. | |||
(c808d2187a73) [_reverted in 0.4.1_] | |||
- Refresh highlighting upon `accept-*` widgets (`accept-line` et al). | |||
(59fbdda64c21) | |||
- Stop leaking match/mbegin/mend to global scope (thanks to upstream | |||
`WARN_CREATE_GLOBAL` improvements). | |||
(d3deffbf46a4) | |||
- 'make install': Permit setting `$(SHARE_DIR)` from the environment. | |||
(e1078a8b4cf1) | |||
- driver: Tolerate KSH_ARRAYS being set in the calling context. | |||
(#162, 8f19af6b319d) | |||
- 'make install': Install documentation fully and properly. | |||
(#219, b1619c001390, et seq) | |||
- docs: Improve 'main' highlighter's documentation. | |||
(00de155063f5, 7d4252f5f596) | |||
- docs: Moved to a new docs/ tree; assorted minor updates | |||
(c575f8f37567, 5b34c23cfad5, et seq) | |||
- docs: Split README.md into INSTALL.md | |||
(0b3183f6cb9a) | |||
- driver: Report `$ZSH_HIGHLIGHT_REVISION` when running from git | |||
(84734ba95026) | |||
## Developer-visible changes: | |||
- Test harness converted to [TAP](http://testanything.org/tap-specification.html) format | |||
(d99aa58aaaef, et seq) | |||
- Run each test in a separate subprocess, isolating them from each other | |||
(d99aa58aaaef, et seq) | |||
- Fix test failure with nonexisting $HOME | |||
(#216, b2ac98b98150) | |||
- Test output is now colorized. | |||
(4d3da30f8b72, 6fe07c096109) | |||
- Document `make install` | |||
(a18a7427fd2c) | |||
- tests: Allow specifying the zsh binary to use. | |||
(557bb7e0c6a0) | |||
- tests: Add 'make perf' target | |||
(4513eaea71d7) | |||
- tests: Run each test in a sandbox directory | |||
(c01533920245) | |||
# Changes in version 0.3.0 | |||
## Added highlighting of: | |||
- suffix aliases (requires zsh 5.1.1 or newer): | |||
```zsh | |||
alias -s png=display | |||
foo.png | |||
``` | |||
- prefix redirections: | |||
```zsh | |||
<foo.txt cat | |||
``` | |||
- redirection operators: | |||
```zsh | |||
echo > foo.txt | |||
``` | |||
- arithmetic evaluations: | |||
```zsh | |||
(( 42 )) | |||
``` | |||
- $'' strings, including \x/\octal/\u/\U escapes | |||
```zsh | |||
: $'foo\u0040bar' | |||
``` | |||
- multiline strings: | |||
```zsh | |||
% echo "line 1 | |||
line 2" | |||
``` | |||
- string literals that haven't been finished: | |||
```zsh | |||
% echo "Hello, world | |||
``` | |||
- command words that involve tilde expansion: | |||
```zsh | |||
% ~/bin/foo | |||
``` | |||
## Fixed highlighting of: | |||
- quoted command words: | |||
```zsh | |||
% \ls | |||
``` | |||
- backslash escapes in "" strings: | |||
```zsh | |||
% echo "\x41" | |||
``` | |||
- noglob after command separator: | |||
```zsh | |||
% :; noglob echo * | |||
``` | |||
- glob after command separator, when the first command starts with 'noglob': | |||
```zsh | |||
% noglob true; echo * | |||
``` | |||
- the region (vi visual mode / set-mark-command) (issue #165) | |||
- redirection and command separators that would be highlighted as `path_approx` | |||
```zsh | |||
% echo foo;‸ | |||
% echo <‸ | |||
``` | |||
(where `‸` represents the cursor location) | |||
- escaped globbing (outside quotes) | |||
```zsh | |||
% echo \* | |||
``` | |||
## Other changes: | |||
- implemented compatibility with zsh's paste highlighting (issue #175) | |||
- `$?` propagated correctly to wrapped widgets | |||
- don't leak $REPLY into global scope | |||
## Developer-visible changes: | |||
- added makefile with `install` and `test` targets | |||
- set `warn_create_global` internally | |||
- document release process | |||
# Version 0.2.1 | |||
(Start of changelog.) | |||
@ -1,132 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters | |||
====================================== | |||
Syntax highlighting is done by pluggable highlighters: | |||
* `main` - the base highlighter, and the only one [active by default][1]. | |||
* `brackets` - [matches brackets][2] and parenthesis. | |||
* `pattern` - matches [user-defined patterns][3]. | |||
* `cursor` - matches [the cursor position][4]. | |||
* `root` - highlights the whole command line [if the current user is root][5]. | |||
* `line` - applied to [the whole command line][6]. | |||
[1]: highlighters/main.md | |||
[2]: highlighters/brackets.md | |||
[3]: highlighters/pattern.md | |||
[4]: highlighters/cursor.md | |||
[5]: highlighters/root.md | |||
[6]: highlighters/line.md | |||
Highlighter-independent settings | |||
-------------------------------- | |||
By default, all command lines are highlighted. However, it is possible to | |||
prevent command lines longer than a fixed number of characters from being | |||
highlighted by setting the variable `${ZSH_HIGHLIGHT_MAXLENGTH}` to the maximum | |||
length (in characters) of command lines to be highlighter. This is useful when | |||
editing very long comand lines (for example, with the [`fned`][fned] utility | |||
function). Example: | |||
[fned]: http://zsh.sourceforge.net/Doc/Release/User-Contributions.html#index-zed | |||
```zsh | |||
ZSH_HIGHLIGHT_MAXLENGTH=512 | |||
``` | |||
How to activate highlighters | |||
---------------------------- | |||
To activate an highlighter, add it to the `ZSH_HIGHLIGHT_HIGHLIGHTERS` array in | |||
`~/.zshrc`, for example: | |||
```zsh | |||
ZSH_HIGHLIGHT_HIGHLIGHTERS=(main brackets pattern cursor) | |||
``` | |||
By default, `$ZSH_HIGHLIGHT_HIGHLIGHTERS` is unset and only the `main` | |||
highlighter is active. | |||
How to tweak highlighters | |||
------------------------- | |||
Highlighters look up styles from the `ZSH_HIGHLIGHT_STYLES` associative array. | |||
Navigate into the [individual highlighters' documentation](highlighters/) to | |||
see what styles (keys) each highlighter defines; the syntax for values is the | |||
same as the syntax of "types of highlighting" of the zsh builtin | |||
`$zle_highlight` array, which is documented in [the `zshzle(1)` manual | |||
page][zshzle-Character-Highlighting]. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting | |||
Some highlighters support additional configuration parameters; see each | |||
highlighter's documentation for details and examples. | |||
How to implement a new highlighter | |||
---------------------------------- | |||
To create your own `acme` highlighter: | |||
* Create your script at | |||
`highlighters/acme/acme-highlighter.zsh`. | |||
* Implement the `_zsh_highlight_highlighter_acme_predicate` function. | |||
This function must return 0 when the highlighter needs to be called and | |||
non-zero otherwise, for example: | |||
```zsh | |||
_zsh_highlight_highlighter_acme_predicate() { | |||
# Call this highlighter in SVN working copies | |||
[[ -d .svn ]] | |||
} | |||
``` | |||
* Implement the `_zsh_highlight_highlighter_acme_paint` function. | |||
This function does the actual syntax highlighting, by calling | |||
`_zsh_highlight_add_highlight` with the start and end of the region to | |||
be highlighted and the `ZSH_HIGHLIGHT_STYLES` key to use. Define the default | |||
style for that key in the highlighter script outside of any function with | |||
`: ${ZSH_HIGHLIGHT_STYLES[key]:=value}`, being sure to prefix | |||
the key with your highlighter name and a colon. For example: | |||
```zsh | |||
: ${ZSH_HIGHLIGHT_STYLES[acme:aurora]:=fg=green} | |||
_zsh_highlight_highlighter_acme_paint() { | |||
# Colorize the whole buffer with the 'aurora' style | |||
_zsh_highlight_add_highlight 0 $#BUFFER acme:aurora | |||
} | |||
``` | |||
If you need to test which options the user has set, test `zsyh_user_options` | |||
with a sensible default if the option is not present in supported zsh | |||
versions. For example: | |||
```zsh | |||
[[ ${zsyh_user_options[ignoreclosebraces]:-off} == on ]] | |||
``` | |||
The option name must be all lowercase with no underscores and not an alias. | |||
* Name your own functions and global variables `_zsh_highlight_acme_*`. | |||
- In zsh-syntax-highlighting 0.4.0 and earlier, the entrypoints | |||
`_zsh_highlight_highlighter_acme_predicate` and | |||
`_zsh_highlight_highlighter_acme_paint` | |||
were named | |||
`_zsh_highlight_acme_highlighter_predicate` and | |||
`_zsh_highlight_highlighter_acme_paint` respectively. | |||
These names are still supported for backwards compatibility; | |||
however, support for them will be removed in a future major or minor release (v0.x.0 or v1.0.0). | |||
* Activate your highlighter in `~/.zshrc`: | |||
```zsh | |||
ZSH_HIGHLIGHT_HIGHLIGHTERS+=(acme) | |||
``` | |||
* [Write tests](../tests/README.md). |
@ -1,31 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / brackets | |||
------------------------------------------------- | |||
This is the `brackets` highlighter, that highlights brackets and parentheses, and | |||
matches them. | |||
### How to tweak it | |||
This highlighter defines the following styles: | |||
* `bracket-error` - unmatched brackets | |||
* `bracket-level-N` - brackets with nest level N | |||
* `cursor-matchingbracket` - the matching bracket, if cursor is on a bracket | |||
To override one of those styles, change its entry in `ZSH_HIGHLIGHT_STYLES`, | |||
for example in `~/.zshrc`: | |||
```zsh | |||
# To define styles for nested brackets up to level 4 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]='fg=blue,bold' | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]='fg=red,bold' | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-3]='fg=yellow,bold' | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-4]='fg=magenta,bold' | |||
``` | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting |
@ -1,24 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / cursor | |||
----------------------------------------------- | |||
This is the `cursor` highlighter, that highlights the cursor. | |||
### How to tweak it | |||
This highlighter defines the following styles: | |||
* `cursor` - the style for the current cursor position | |||
To override one of those styles, change its entry in `ZSH_HIGHLIGHT_STYLES`, | |||
for example in `~/.zshrc`: | |||
```zsh | |||
ZSH_HIGHLIGHT_STYLES[cursor]='bg=blue' | |||
``` | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting |
@ -1,24 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / line | |||
--------------------------------------------- | |||
This is the `line` highlighter, that highlights the whole line. | |||
### How to tweak it | |||
This highlighter defines the following styles: | |||
* `line` - the style for the whole line | |||
To override one of those styles, change its entry in `ZSH_HIGHLIGHT_STYLES`, | |||
for example in `~/.zshrc`: | |||
```zsh | |||
ZSH_HIGHLIGHT_STYLES[line]='bold' | |||
``` | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting |
@ -1,121 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / main | |||
--------------------------------------------- | |||
This is the `main` highlighter, that highlights: | |||
* Commands | |||
* Options | |||
* Arguments | |||
* Paths | |||
* Strings | |||
This highlighter is active by default. | |||
### How to tweak it | |||
This highlighter defines the following styles: | |||
* `unknown-token` - unknown tokens / errors | |||
* `reserved-word` - shell reserved words (`if`, `for`) | |||
* `alias` - aliases | |||
* `suffix-alias` - suffix aliases (requires zsh 5.1.1 or newer) | |||
* `global-alias` - global aliases | |||
* `builtin` - shell builtin commands (`shift`, `pwd`, `zstyle`) | |||
* `function` - function names | |||
* `command` - command names | |||
* `precommand` - precommand modifiers (e.g., `noglob`, `builtin`) | |||
* `commandseparator` - command separation tokens (`;`, `&&`) | |||
* `hashed-command` - hashed commands | |||
* `autodirectory` - a directory name in command position when the `AUTO_CD` option is set | |||
* `path` - existing filenames | |||
* `path_pathseparator` - path separators in filenames (`/`); if unset, `path` is used (default) | |||
* `path_prefix` - prefixes of existing filenames | |||
* `path_prefix_pathseparator` - path separators in prefixes of existing filenames (`/`); if unset, `path_prefix` is used (default) | |||
* `globbing` - globbing expressions (`*.txt`) | |||
* `history-expansion` - history expansion expressions (`!foo` and `^foo^bar`) | |||
* `command-substitution` - command substitutions (`$(echo foo)`) | |||
* `command-substitution-unquoted` - an unquoted command substitution (`$(echo foo)`) | |||
* `command-substitution-quoted` - a quoted command substitution (`"$(echo foo)"`) | |||
* `command-substitution-delimiter` - command substitution delimiters (`$(` and `)`) | |||
* `command-substitution-delimiter-unquoted` - an unquoted command substitution delimiters (`$(` and `)`) | |||
* `command-substitution-delimiter-quoted` - a quoted command substitution delimiters (`"$(` and `)"`) | |||
* `process-substitution` - process substitutions (`<(echo foo)`) | |||
* `process-substitution-delimiter` - process substitution delimiters (`<(` and `)`) | |||
* `arithmetic-expansion` - arithmetic expansion `$(( 42 ))`) | |||
* `single-hyphen-option` - single-hyphen options (`-o`) | |||
* `double-hyphen-option` - double-hyphen options (`--option`) | |||
* `back-quoted-argument` - backtick command substitution (`` `foo` ``) | |||
* `back-quoted-argument-unclosed` - unclosed backtick command substitution (`` `foo ``) | |||
* `back-quoted-argument-delimiter` - backtick command substitution delimiters (`` ` ``) | |||
* `single-quoted-argument` - single-quoted arguments (`` 'foo' ``) | |||
* `single-quoted-argument-unclosed` - unclosed single-quoted arguments (`` 'foo ``) | |||
* `double-quoted-argument` - double-quoted arguments (`` "foo" ``) | |||
* `double-quoted-argument-unclosed` - unclosed double-quoted arguments (`` "foo ``) | |||
* `dollar-quoted-argument` - dollar-quoted arguments (`` $'foo' ``) | |||
* `dollar-quoted-argument-unclosed` - unclosed dollar-quoted arguments (`` $'foo ``) | |||
* `rc-quote` - two single quotes inside single quotes when the `RC_QUOTES` option is set (`` 'foo''bar' ``) | |||
* `dollar-double-quoted-argument` - parameter expansion inside double quotes (`$foo` inside `""`) | |||
* `back-double-quoted-argument` - backslash escape sequences inside double-quoted arguments (`\"` in `"foo\"bar"`) | |||
* `back-dollar-quoted-argument` - backslash escape sequences inside dollar-quoted arguments (`\x` in `$'\x48'`) | |||
* `assign` - parameter assignments (`x=foo` and `x=( )`) | |||
* `redirection` - redirection operators (`<`, `>`, etc) | |||
* `comment` - comments, when `setopt INTERACTIVE_COMMENTS` is in effect (`echo # foo`) | |||
* `comment` - elided parameters in command position (`$x ls` when `$x` is unset or empty) | |||
* `named-fd` - named file descriptor (the `fd` in `echo foo {fd}>&2`) | |||
* `numeric-fd` - numeric file descriptor (the `2` in `echo foo {fd}>&2`) | |||
* `arg0` - a command word other than one of those enumerated above (other than a command, precommand, alias, function, or shell builtin command). | |||
* `default` - everything else | |||
To override one of those styles, change its entry in `ZSH_HIGHLIGHT_STYLES`, | |||
for example in `~/.zshrc`: | |||
```zsh | |||
# Declare the variable | |||
typeset -A ZSH_HIGHLIGHT_STYLES | |||
# To differentiate aliases from other command types | |||
ZSH_HIGHLIGHT_STYLES[alias]='fg=magenta,bold' | |||
# To have paths colored instead of underlined | |||
ZSH_HIGHLIGHT_STYLES[path]='fg=cyan' | |||
# To disable highlighting of globbing expressions | |||
ZSH_HIGHLIGHT_STYLES[globbing]='none' | |||
``` | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
#### Parameters | |||
To avoid partial path lookups on a path, add the path to the `ZSH_HIGHLIGHT_DIRS_BLACKLIST` array. | |||
```zsh | |||
ZSH_HIGHLIGHT_DIRS_BLACKLIST+=(/mnt/slow_share) | |||
``` | |||
### Useless trivia | |||
#### Forward compatibility. | |||
zsh-syntax-highlighting attempts to be forward-compatible with zsh. | |||
Specifically, we attempt to facilitate highlighting _command word_ types that | |||
had not yet been invented when this version of zsh-syntax-highlighting was | |||
released. | |||
A _command word_ is something like a function name, external command name, et | |||
cetera. (See | |||
[Simple Commands & Pipelines in `zshmisc(1)`][zshmisc-Simple-Commands-And-Pipelines] | |||
for a formal definition.) | |||
If a new _kind_ of command word is ever added to zsh — something conceptually | |||
different than "function" and "alias" and "external command" — then command words | |||
of that (new) kind will be highlighted by the style `arg0_$kind`, | |||
where `$kind` is the output of `type -w` on the new kind of command word. If that | |||
style is not defined, then the style `arg0` will be used instead. | |||
[zshmisc-Simple-Commands-And-Pipelines]: http://zsh.sourceforge.net/Doc/Release/Shell-Grammar.html#Simple-Commands-_0026-Pipelines | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting |
@ -1,24 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / pattern | |||
------------------------------------------------ | |||
This is the `pattern` highlighter, that highlights user-defined patterns. | |||
### How to tweak it | |||
To use this highlighter, associate patterns with styles in the | |||
`ZSH_HIGHLIGHT_PATTERNS` associative array, for example in `~/.zshrc`: | |||
```zsh | |||
# Declare the variable | |||
typeset -A ZSH_HIGHLIGHT_PATTERNS | |||
# To have commands starting with `rm -rf` in red: | |||
ZSH_HIGHLIGHT_PATTERNS+=('rm -rf *' 'fg=white,bold,bg=red') | |||
``` | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting |
@ -1,30 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / regexp | |||
------------------------------------------------ | |||
This is the `regexp` highlighter, that highlights user-defined regular | |||
expressions. It's similar to the `pattern` highlighter, but allows more complex | |||
patterns. | |||
### How to tweak it | |||
To use this highlighter, associate regular expressions with styles in the | |||
`ZSH_HIGHLIGHT_REGEXP` associative array, for example in `~/.zshrc`: | |||
```zsh | |||
typeset -A ZSH_HIGHLIGHT_REGEXP | |||
ZSH_HIGHLIGHT_REGEXP+=('\bsudo\b' fg=123,bold) | |||
``` | |||
This will highlight "sudo" only as a complete word, i.e., "sudo cmd", but not | |||
"sudoedit" | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
See also: [regular expressions tutorial][perlretut], zsh regexp operator `=~` | |||
in [the `zshmisc(1)` manual page][zshmisc-Conditional-Expressions] | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting | |||
[perlretut]: http://perldoc.perl.org/perlretut.html | |||
[zshmisc-Conditional-Expressions]: http://zsh.sourceforge.net/Doc/Release/Conditional-Expressions.html#Conditional-Expressions |
@ -1,25 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters / root | |||
--------------------------------------------- | |||
This is the `root` highlighter, that highlights the whole line if the current | |||
user is root. | |||
### How to tweak it | |||
This highlighter defines the following styles: | |||
* `root` - the style for the whole line if the current user is root. | |||
To override one of those styles, change its entry in `ZSH_HIGHLIGHT_STYLES`, | |||
for example in `~/.zshrc`: | |||
```zsh | |||
ZSH_HIGHLIGHT_STYLES[root]='bg=red' | |||
``` | |||
The syntax for values is the same as the syntax of "types of highlighting" of | |||
the zsh builtin `$zle_highlight` array, which is documented in [the `zshzle(1)` | |||
manual page][zshzle-Character-Highlighting]. | |||
[zshzle-Character-Highlighting]: http://zsh.sourceforge.net/Doc/Release/Zsh-Line-Editor.html#Character-Highlighting |
@ -0,0 +1,77 @@ | |||
# vim:ft=zsh:sw=4:sts=4 | |||
# | |||
# $1 - PREBUFFER | |||
# $2 - BUFFER | |||
# | |||
function -fast-highlight-string-process { | |||
emulate -LR zsh | |||
setopt extendedglob warncreateglobal typesetsilent | |||
local -A pos_to_level level_to_pos pair_map final_pairs | |||
local input=$1$2 _mybuf=$1$2 __style __quoting | |||
integer __idx=0 __pair_idx __level=0 __start __end | |||
local -a match mbegin mend | |||
pair_map=( "(" ")" "{" "}" "[" "]" ) | |||
while [[ $_mybuf = (#b)([^"{}()[]\\\"'"]#)((["({[]})\"'"])|[\\](*))(*) ]]; do | |||
if [[ -n ${match[4]} ]] { | |||
__idx+=${mbegin[2]} | |||
[[ $__quoting = \' ]] && _mybuf=${match[4]} || { _mybuf=${match[4]:1}; (( ++ __idx )); } | |||
} else { | |||
__idx+=${mbegin[2]} | |||
[[ -z $__quoting && -z ${_FAST_COMPLEX_BRACKETS[(r)$((__idx-${#PREBUFFER}-1))]} ]] && { | |||
if [[ ${match[2]} = ["({["] ]]; then | |||
pos_to_level[$__idx]=$(( ++__level )) | |||
level_to_pos[$__level]=$__idx | |||
elif [[ ${match[2]} = ["]})"] ]]; then | |||
if (( __level > 0 )); then | |||
__pair_idx=${level_to_pos[$__level]} | |||
pos_to_level[$__idx]=$(( __level -- )) | |||
[[ ${pair_map[${input[__pair_idx]}]} = ${input[__idx]} ]] && { | |||
final_pairs[$__idx]=$__pair_idx | |||
final_pairs[$__pair_idx]=$__idx | |||
} | |||
else | |||
pos_to_level[$__idx]=-1 | |||
fi | |||
fi | |||
} | |||
if [[ ${match[2]} = \" && $__quoting != \' ]] { | |||
[[ $__quoting = '"' ]] && __quoting="" || __quoting='"'; | |||
} | |||
if [[ ${match[2]} = \' && $__quoting != \" ]] { | |||
if [[ $__quoting = ("'"|"$'") ]] { | |||
__quoting="" | |||
} else { | |||
if [[ $match[1] = *\$ ]] { | |||
__quoting="\$'"; | |||
} else { | |||
__quoting="'"; | |||
} | |||
} | |||
} | |||
_mybuf=${match[5]} | |||
} | |||
done | |||
for __idx in ${(k)pos_to_level}; do | |||
(( ${+final_pairs[$__idx]} )) && __style=${FAST_THEME_NAME}bracket-level-$(( ( (pos_to_level[$__idx]-1) % 3 ) + 1 )) || __style=${FAST_THEME_NAME}unknown-token | |||
(( __start=__idx-${#PREBUFFER}-1, __end=__idx-${#PREBUFFER}, __start >= 0 )) && \ | |||
reply+=("$__start $__end ${FAST_HIGHLIGHT_STYLES[$__style]}") | |||
done | |||
# If cursor is on a bracket, then highlight corresponding bracket, if any. | |||
if [[ $WIDGET != zle-line-finish ]]; then | |||
__idx=$(( CURSOR + 1 )) | |||
if (( ${+pos_to_level[$__idx]} )) && (( ${+final_pairs[$__idx]} )); then | |||
(( __start=final_pairs[$__idx]-${#PREBUFFER}-1, __end=final_pairs[$__idx]-${#PREBUFFER}, __start >= 0 )) && \ | |||
reply+=("$__start $__end ${FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}paired-bracket]}") && \ | |||
reply+=("$CURSOR $__idx ${FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}paired-bracket]}") | |||
fi | |||
fi | |||
return 0 | |||
} |
@ -0,0 +1,384 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2010-2016 zsh-syntax-highlighting contributors | |||
# Copyright (c) 2017-2019 Sebastian Gniazdowski (modifications) | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Standarized way of handling finding plugin dir, | |||
# regardless of functionargzero and posixargzero, | |||
# and with an option for a plugin manager to alter | |||
# the plugin directory (i.e. set ZERO parameter) | |||
# http://zdharma.org/Zsh-100-Commits-Club/Zsh-Plugin-Standard.html | |||
0="${${ZERO:-${0:#$ZSH_ARGZERO}}:-${(%):-%N}}" | |||
0="${${(M)0:#/*}:-$PWD/$0}" | |||
typeset -g FAST_HIGHLIGHT_VERSION=1.55 | |||
typeset -g FAST_BASE_DIR="${0:h}" | |||
typeset -ga _FAST_MAIN_CACHE | |||
# Holds list of indices pointing at brackets that | |||
# are complex, i.e. e.g. part of "[[" in [[ ... ]] | |||
typeset -ga _FAST_COMPLEX_BRACKETS | |||
typeset -g FAST_WORK_DIR=${FAST_WORK_DIR:-${XDG_CACHE_HOME:-~/.cache}/fast-syntax-highlighting} | |||
: ${FAST_WORK_DIR:=$FAST_BASE_DIR} | |||
# Expand any tilde in the (supposed) path. | |||
FAST_WORK_DIR=${~FAST_WORK_DIR} | |||
# Last (currently, possibly) loaded plugin isn't "fast-syntax-highlighting"? | |||
# And FPATH isn't containing plugin dir? | |||
if [[ ${zsh_loaded_plugins[-1]} != */fast-syntax-highlighting && -z ${fpath[(r)${0:h}]} ]] | |||
then | |||
fpath+=( "${0:h}" ) | |||
fi | |||
if [[ ! -w $FAST_WORK_DIR ]]; then | |||
FAST_WORK_DIR="${XDG_CACHE_HOME:-$HOME/.cache}/fsh" | |||
command mkdir -p "$FAST_WORK_DIR" | |||
fi | |||
# Invokes each highlighter that needs updating. | |||
# This function is supposed to be called whenever the ZLE state changes. | |||
_zsh_highlight() | |||
{ | |||
# Store the previous command return code to restore it whatever happens. | |||
local ret=$? | |||
# Remove all highlighting in isearch, so that only the underlining done by zsh itself remains. | |||
# For details see FAQ entry 'Why does syntax highlighting not work while searching history?'. | |||
if [[ $WIDGET == zle-isearch-update ]] && ! (( $+ISEARCHMATCH_ACTIVE )); then | |||
region_highlight=() | |||
return $ret | |||
fi | |||
emulate -LR zsh | |||
setopt extendedglob warncreateglobal typesetsilent noshortloops | |||
local REPLY # don't leak $REPLY into global scope | |||
local -a reply | |||
# Do not highlight if there are more than 300 chars in the buffer. It's most | |||
# likely a pasted command or a huge list of files in that case.. | |||
[[ -n ${ZSH_HIGHLIGHT_MAXLENGTH:-} ]] && [[ $#BUFFER -gt $ZSH_HIGHLIGHT_MAXLENGTH ]] && return $ret | |||
# Do not highlight if there are pending inputs (copy/paste). | |||
[[ $PENDING -gt 0 ]] && return $ret | |||
# Reset region highlight to build it from scratch | |||
# may need to remove path_prefix highlighting when the line ends | |||
if [[ $WIDGET == zle-line-finish ]] || _zsh_highlight_buffer_modified; then | |||
-fast-highlight-init | |||
-fast-highlight-process "$PREBUFFER" "$BUFFER" 0 | |||
(( FAST_HIGHLIGHT[use_brackets] )) && { | |||
_FAST_MAIN_CACHE=( $reply ) | |||
-fast-highlight-string-process "$PREBUFFER" "$BUFFER" | |||
} | |||
region_highlight=( $reply ) | |||
else | |||
local char="${BUFFER[CURSOR+1]}" | |||
if [[ "$char" = ["{([])}"] || "${FAST_HIGHLIGHT[prev_char]}" = ["{([])}"] ]]; then | |||
FAST_HIGHLIGHT[prev_char]="$char" | |||
(( FAST_HIGHLIGHT[use_brackets] )) && { | |||
reply=( $_FAST_MAIN_CACHE ) | |||
-fast-highlight-string-process "$PREBUFFER" "$BUFFER" | |||
region_highlight=( $reply ) | |||
} | |||
fi | |||
fi | |||
{ | |||
local cache_place | |||
local -a region_highlight_copy | |||
# Re-apply zle_highlight settings | |||
# region | |||
if (( REGION_ACTIVE == 1 )); then | |||
_zsh_highlight_apply_zle_highlight region standout "$MARK" "$CURSOR" | |||
elif (( REGION_ACTIVE == 2 )); then | |||
() { | |||
local needle=$'\n' | |||
integer min max | |||
if (( MARK > CURSOR )) ; then | |||
min=$CURSOR max=$(( MARK + 1 )) | |||
else | |||
min=$MARK max=$CURSOR | |||
fi | |||
(( min = ${${BUFFER[1,$min]}[(I)$needle]} )) | |||
(( max += ${${BUFFER:($max-1)}[(i)$needle]} - 1 )) | |||
_zsh_highlight_apply_zle_highlight region standout "$min" "$max" | |||
} | |||
fi | |||
# yank / paste (zsh-5.1.1 and newer) | |||
(( $+YANK_ACTIVE )) && (( YANK_ACTIVE )) && _zsh_highlight_apply_zle_highlight paste standout "$YANK_START" "$YANK_END" | |||
# isearch | |||
(( $+ISEARCHMATCH_ACTIVE )) && (( ISEARCHMATCH_ACTIVE )) && _zsh_highlight_apply_zle_highlight isearch underline "$ISEARCHMATCH_START" "$ISEARCHMATCH_END" | |||
# suffix | |||
(( $+SUFFIX_ACTIVE )) && (( SUFFIX_ACTIVE )) && _zsh_highlight_apply_zle_highlight suffix bold "$SUFFIX_START" "$SUFFIX_END" | |||
return $ret | |||
} always { | |||
typeset -g _ZSH_HIGHLIGHT_PRIOR_BUFFER="$BUFFER" | |||
typeset -g _ZSH_HIGHLIGHT_PRIOR_RACTIVE="$REGION_ACTIVE" | |||
typeset -gi _ZSH_HIGHLIGHT_PRIOR_CURSOR=$CURSOR | |||
} | |||
} | |||
# Apply highlighting based on entries in the zle_highlight array. | |||
# This function takes four arguments: | |||
# 1. The exact entry (no patterns) in the zle_highlight array: | |||
# region, paste, isearch, or suffix | |||
# 2. The default highlighting that should be applied if the entry is unset | |||
# 3. and 4. Two integer values describing the beginning and end of the | |||
# range. The order does not matter. | |||
_zsh_highlight_apply_zle_highlight() { | |||
local entry="$1" default="$2" | |||
integer first="$3" second="$4" | |||
# read the relevant entry from zle_highlight | |||
local region="${zle_highlight[(r)${entry}:*]}" | |||
if [[ -z "$region" ]]; then | |||
# entry not specified at all, use default value | |||
region=$default | |||
else | |||
# strip prefix | |||
region="${region#${entry}:}" | |||
# no highlighting when set to the empty string or to 'none' | |||
if [[ -z "$region" ]] || [[ "$region" == none ]]; then | |||
return | |||
fi | |||
fi | |||
integer start end | |||
if (( first < second )); then | |||
start=$first end=$second | |||
else | |||
start=$second end=$first | |||
fi | |||
region_highlight+=("$start $end $region") | |||
} | |||
# ------------------------------------------------------------------------------------------------- | |||
# API/utility functions for highlighters | |||
# ------------------------------------------------------------------------------------------------- | |||
# Whether the command line buffer has been modified or not. | |||
# | |||
# Returns 0 if the buffer has changed since _zsh_highlight was last called. | |||
_zsh_highlight_buffer_modified() | |||
{ | |||
[[ "${_ZSH_HIGHLIGHT_PRIOR_BUFFER:-}" != "$BUFFER" ]] || [[ "$REGION_ACTIVE" != "$_ZSH_HIGHLIGHT_PRIOR_RACTIVE" ]] || { _zsh_highlight_cursor_moved && [[ "$REGION_ACTIVE" = 1 || "$REGION_ACTIVE" = 2 ]] } | |||
} | |||
# Whether the cursor has moved or not. | |||
# | |||
# Returns 0 if the cursor has moved since _zsh_highlight was last called. | |||
_zsh_highlight_cursor_moved() | |||
{ | |||
[[ -n $CURSOR ]] && [[ -n ${_ZSH_HIGHLIGHT_PRIOR_CURSOR-} ]] && (($_ZSH_HIGHLIGHT_PRIOR_CURSOR != $CURSOR)) | |||
} | |||
# ------------------------------------------------------------------------------------------------- | |||
# Setup functions | |||
# ------------------------------------------------------------------------------------------------- | |||
# Helper for _zsh_highlight_bind_widgets | |||
# $1 is name of widget to call | |||
_zsh_highlight_call_widget() | |||
{ | |||
integer ret | |||
builtin zle "$@" | |||
ret=$? | |||
_zsh_highlight | |||
return $ret | |||
} | |||
# Rebind all ZLE widgets to make them invoke _zsh_highlights. | |||
_zsh_highlight_bind_widgets() | |||
{ | |||
setopt localoptions noksharrays | |||
local -F2 SECONDS | |||
local prefix=orig-s${SECONDS/./}-r$(( RANDOM % 1000 )) # unique each time, in case we're sourced more than once | |||
# Load ZSH module zsh/zleparameter, needed to override user defined widgets. | |||
zmodload zsh/zleparameter 2>/dev/null || { | |||
print -r -- >&2 'zsh-syntax-highlighting: failed loading zsh/zleparameter.' | |||
return 1 | |||
} | |||
# Override ZLE widgets to make them invoke _zsh_highlight. | |||
local -U widgets_to_bind | |||
widgets_to_bind=(${${(k)widgets}:#(.*|run-help|which-command|beep|set-local-history|yank|zle-line-pre-redraw|zle-keymap-select)}) | |||
# Always wrap special zle-line-finish widget. This is needed to decide if the | |||
# current line ends and special highlighting logic needs to be applied. | |||
# E.g. remove cursor imprint, don't highlight partial paths, ... | |||
widgets_to_bind+=(zle-line-finish) | |||
# Always wrap special zle-isearch-update widget to be notified of updates in isearch. | |||
# This is needed because we need to disable highlighting in that case. | |||
widgets_to_bind+=(zle-isearch-update) | |||
local cur_widget | |||
for cur_widget in $widgets_to_bind; do | |||
case $widgets[$cur_widget] in | |||
# Already rebound event: do nothing. | |||
user:_zsh_highlight_widget_*);; | |||
# The "eval"'s are required to make $cur_widget a closure: the value of the parameter at function | |||
# definition time is used. | |||
# | |||
# We can't use ${0/_zsh_highlight_widget_} because these widgets are always invoked with | |||
# NO_function_argzero, regardless of the option's setting here. | |||
# User defined widget: override and rebind old one with prefix "orig-". | |||
user:*) zle -N -- $prefix-$cur_widget ${widgets[$cur_widget]#*:} | |||
eval "_zsh_highlight_widget_${(q)prefix}-${(q)cur_widget}() { _zsh_highlight_call_widget ${(q)prefix}-${(q)cur_widget} -- \"\$@\" }" | |||
zle -N -- $cur_widget _zsh_highlight_widget_$prefix-$cur_widget;; | |||
# Completion widget: override and rebind old one with prefix "orig-". | |||
completion:*) zle -C $prefix-$cur_widget ${${(s.:.)widgets[$cur_widget]}[2,3]} | |||
eval "_zsh_highlight_widget_${(q)prefix}-${(q)cur_widget}() { _zsh_highlight_call_widget ${(q)prefix}-${(q)cur_widget} -- \"\$@\" }" | |||
zle -N -- $cur_widget _zsh_highlight_widget_$prefix-$cur_widget;; | |||
# Builtin widget: override and make it call the builtin ".widget". | |||
builtin) eval "_zsh_highlight_widget_${(q)prefix}-${(q)cur_widget}() { _zsh_highlight_call_widget .${(q)cur_widget} -- \"\$@\" }" | |||
zle -N -- $cur_widget _zsh_highlight_widget_$prefix-$cur_widget;; | |||
# Incomplete or nonexistent widget: Bind to z-sy-h directly. | |||
*) | |||
if [[ $cur_widget == zle-* ]] && [[ -z $widgets[$cur_widget] ]]; then | |||
_zsh_highlight_widget_${cur_widget}() { :; _zsh_highlight } | |||
zle -N -- $cur_widget _zsh_highlight_widget_$cur_widget | |||
else | |||
# Default: unhandled case. | |||
print -r -- >&2 "zsh-syntax-highlighting: unhandled ZLE widget ${(qq)cur_widget}" | |||
fi | |||
esac | |||
done | |||
} | |||
# ------------------------------------------------------------------------------------------------- | |||
# Setup | |||
# ------------------------------------------------------------------------------------------------- | |||
# Try binding widgets. | |||
_zsh_highlight_bind_widgets || { | |||
print -r -- >&2 'zsh-syntax-highlighting: failed binding ZLE widgets, exiting.' | |||
return 1 | |||
} | |||
# Reset scratch variables when commandline is done. | |||
_zsh_highlight_preexec_hook() | |||
{ | |||
typeset -g _ZSH_HIGHLIGHT_PRIOR_BUFFER= | |||
typeset -gi _ZSH_HIGHLIGHT_PRIOR_CURSOR=0 | |||
typeset -ga _FAST_MAIN_CACHE | |||
_FAST_MAIN_CACHE=() | |||
} | |||
autoload -Uz add-zsh-hook | |||
add-zsh-hook preexec _zsh_highlight_preexec_hook 2>/dev/null || { | |||
print -r -- >&2 'zsh-syntax-highlighting: failed loading add-zsh-hook.' | |||
} | |||
/fshdbg() { | |||
print -r -- "$@" >>! /tmp/reply | |||
} | |||
ZSH_HIGHLIGHT_MAXLENGTH=10000 | |||
# Load zsh/parameter module if available | |||
zmodload zsh/parameter 2>/dev/null | |||
zmodload zsh/system 2>/dev/null | |||
autoload -Uz -- is-at-least fast-theme .fast-read-ini-file .fast-run-git-command \ | |||
.fast-make-targets .fast-run-command .fast-zts-read-all | |||
autoload -Uz -- →chroma/-git.ch →chroma/-hub.ch →chroma/-lab.ch →chroma/-example.ch \ | |||
→chroma/-grep.ch →chroma/-perl.ch →chroma/-make.ch →chroma/-awk.ch \ | |||
→chroma/-vim.ch →chroma/-source.ch →chroma/-sh.ch →chroma/-docker.ch \ | |||
→chroma/-autoload.ch →chroma/-ssh.ch →chroma/-scp.ch →chroma/-which.ch \ | |||
→chroma/-printf.ch →chroma/-ruby.ch →chroma/-whatis.ch →chroma/-alias.ch \ | |||
→chroma/-subcommand.ch →chroma/-autorandr.ch →chroma/-nmcli.ch \ | |||
→chroma/-fast-theme.ch →chroma/-node.ch →chroma/-fpath_peq.ch \ | |||
→chroma/-precommand.ch →chroma/-subversion.ch →chroma/-ionice.ch \ | |||
→chroma/-nice.ch →chroma/main-chroma.ch →chroma/-ogit.ch →chroma/-zinit.ch | |||
source "${0:h}/fast-highlight" | |||
source "${0:h}/fast-string-highlight" | |||
local __fsyh_theme | |||
zstyle -s :plugin:fast-syntax-highlighting theme __fsyh_theme | |||
[[ ( "${+termcap}" != 1 || "${termcap[Co]}" != <-> || "${termcap[Co]}" -lt "256" ) && "$__fsyh_theme" = (default|) ]] && { | |||
FAST_HIGHLIGHT_STYLES[defaultvariable]="none" | |||
FAST_HIGHLIGHT_STYLES[defaultglobbing-ext]="fg=blue,bold" | |||
FAST_HIGHLIGHT_STYLES[defaulthere-string-text]="bg=blue" | |||
FAST_HIGHLIGHT_STYLES[defaulthere-string-var]="fg=cyan,bg=blue" | |||
FAST_HIGHLIGHT_STYLES[defaultcorrect-subtle]="bg=blue" | |||
FAST_HIGHLIGHT_STYLES[defaultsubtle-bg]="bg=blue" | |||
[[ "${FAST_HIGHLIGHT_STYLES[variable]}" = "fg=113" ]] && FAST_HIGHLIGHT_STYLES[variable]="none" | |||
[[ "${FAST_HIGHLIGHT_STYLES[globbing-ext]}" = "fg=13" ]] && FAST_HIGHLIGHT_STYLES[globbing-ext]="fg=blue,bold" | |||
[[ "${FAST_HIGHLIGHT_STYLES[here-string-text]}" = "bg=18" ]] && FAST_HIGHLIGHT_STYLES[here-string-text]="bg=blue" | |||
[[ "${FAST_HIGHLIGHT_STYLES[here-string-var]}" = "fg=cyan,bg=18" ]] && FAST_HIGHLIGHT_STYLES[here-string-var]="fg=cyan,bg=blue" | |||
[[ "${FAST_HIGHLIGHT_STYLES[correct-subtle]}" = "fg=12" ]] && FAST_HIGHLIGHT_STYLES[correct-subtle]="bg=blue" | |||
[[ "${FAST_HIGHLIGHT_STYLES[subtle-bg]}" = "bg=18" ]] && FAST_HIGHLIGHT_STYLES[subtle-bg]="bg=blue" | |||
} | |||
unset __fsyh_theme | |||
alias fsh-alias=fast-theme | |||
-fast-highlight-fill-option-variables | |||
if [[ ! -e $FAST_WORK_DIR/secondary_theme.zsh ]] { | |||
if { type curl &>/dev/null } { | |||
curl -fsSL -o "$FAST_WORK_DIR/secondary_theme.zsh" \ | |||
https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/share/free_theme.zsh \ | |||
&>/dev/null | |||
} elif { type wget &>/dev/null } { | |||
wget -O "$FAST_WORK_DIR/secondary_theme.zsh" \ | |||
https://raw.githubusercontent.com/zdharma/fast-syntax-highlighting/master/share/free_theme.zsh \ | |||
&>/dev/null | |||
} | |||
touch "$FAST_WORK_DIR/secondary_theme.zsh" | |||
} | |||
if [[ $(uname -a) = (#i)*darwin* ]] { | |||
typeset -gA FAST_HIGHLIGHT | |||
FAST_HIGHLIGHT[chroma-man]= | |||
} | |||
[[ $COLORTERM == (24bit|truecolor) || ${terminfo[colors]} -eq 16777216 ]] || zmodload zsh/nearcolor &>/dev/null |
@ -0,0 +1,385 @@ | |||
# -*- mode: sh; sh-indentation: 4; indent-tabs-mode: nil; sh-basic-offset: 4; -*- | |||
# Copyright (c) 2018 Sebastian Gniazdowski | |||
# Copyright (c) 2018, 2019 Philippe Troin (F-i-f on GitHub) | |||
# | |||
# Theme support using ini-files. | |||
# | |||
zmodload zsh/zutil 2>/dev/null | |||
emulate -LR zsh | |||
setopt extendedglob typesetsilent warncreateglobal | |||
autoload colors; colors | |||
typeset -g FAST_WORK_DIR | |||
: ${FAST_WORK_DIR:=$FAST_BASE_DIR} | |||
FAST_WORK_DIR=${~FAST_WORK_DIR} | |||
local -A map | |||
map=( "XDG:" "${XDG_CONFIG_HOME:-$HOME/.config}/fsh/" | |||
"LOCAL:" "/usr/local/share/fsh/" | |||
"HOME:" "$HOME/.fsh/" | |||
"OPT:" "/opt/local/share/fsh/" | |||
) | |||
FAST_WORK_DIR=${${FAST_WORK_DIR/(#m)(#s)(XDG|LOCAL|HOME|OPT):(#c0,1)/${map[${MATCH%:}:]}}%/} | |||
local OPT_HELP OPT_VERBOSE OPT_QUIET OPT_RESET OPT_LIST OPT_TEST OPT_SECONDARY OPT_SHOW OPT_COPY OPT_OV_RESET | |||
local OPT_PALETTE OPT_CDWD OPT_XCHG OPT_OV_XCHG | |||
local -A opthash | |||
zparseopts -E -D -A opthash h -help v -verbose q -quiet r -reset l -list t -test -secondary \ | |||
s -show -copy-shipped-theme: R -ov-reset p -palette w -workdir \ | |||
x -xchg y -ov-xchg || \ | |||
{ echo "Improper options given, see help (-h/--help)"; return 1; } | |||
(( ${+opthash[-h]} + ${+opthash[--help]} )) && OPT_HELP="-h" | |||
(( ${+opthash[-v]} + ${+opthash[--verbose]} )) && OPT_VERBOSE="-v" | |||
(( ${+opthash[-q]} + ${+opthash[--quiet]} )) && OPT_QUIET="-q" | |||
(( ${+opthash[-r]} + ${+opthash[--reset]} )) && OPT_RESET="-r" | |||
(( ${+opthash[-l]} + ${+opthash[--list]} )) && OPT_LIST="-l" | |||
(( ${+opthash[-t]} + ${+opthash[--test]} )) && OPT_TEST="-t" | |||
(( ${+opthash[--secondary]} )) && OPT_SECONDARY="--secondary" | |||
(( ${+opthash[-s]} + ${+opthash[--show]} )) && OPT_SHOW="-s" | |||
(( ${+opthash[--copy-shipped-theme]} )) && OPT_COPY="${opthash[--copy-shipped-theme]}" | |||
(( ${+opthash[-R]} + ${+opthash[--ov-reset]} )) && OPT_OV_RESET="-R" | |||
(( ${+opthash[-p]} + ${+opthash[--palette]} )) && OPT_PALETTE="-p" | |||
(( ${+opthash[-w]} + ${+opthash[--workdir]} )) && OPT_CDWD="-w" | |||
(( ${+opthash[-x]} + ${+opthash[--xchg]} )) && OPT_XCHG="-x" | |||
(( ${+opthash[-y]} + ${+opthash[--ov-xchg]} )) && OPT_OV_XCHG="-y" | |||
local -a match mbegin mend | |||
local MATCH; integer MBEGIN MEND | |||
[[ -n "$OPT_CDWD" ]] && { | |||
builtin cd $FAST_WORK_DIR | |||
return 0 | |||
} | |||
[[ -n "$OPT_PALETTE" ]] && { | |||
local n | |||
local -a __colors | |||
for n in {000..255} | |||
do | |||
__colors+=("%F{$n}$n%f") | |||
done | |||
print -cP $__colors | |||
return | |||
} | |||
[[ -n "$OPT_SHOW" ]] && { | |||
print -r -- "Currently active theme: ${fg_bold[yellow]}$FAST_THEME_NAME$reset_color" | |||
( source "$FAST_WORK_DIR"/current_theme.zsh 2>/dev/null && print "Main theme (loaded at startup of a session): ${fg_bold[yellow]}$FAST_THEME_NAME$reset_color" || print "No main theme is set"; ) | |||
return 0 | |||
} | |||
[[ -n "$OPT_COPY" ]] && { | |||
[[ ! -f "$FAST_BASE_DIR"/themes/"${OPT_COPY%.ini}.ini" ]] && { print "Theme \`$OPT_COPY' doesn't exist in FSH plugin dir ($FAST_BASE_DIR/themes)"; return 1; } | |||
[[ ! -r "$FAST_BASE_DIR"/themes/"${OPT_COPY%.ini}.ini" ]] && { print "Theme \`$OPT_COPY' isn't readable in FSH plugin dir ($FAST_BASE_DIR/themes)"; return 1; } | |||
[[ -n "$1" ]] && { | |||
[[ ! -e "$1" && ! -e ${1:h} ]] && { print "Destination path doesn't exist, aborting"; return 1; } | |||
} | |||
command cp -vf "$FAST_BASE_DIR"/themes/"${OPT_COPY%.ini}.ini" "${${1:-.}%.ini}.ini" || return 1 | |||
return 0 | |||
} | |||
[[ -n "$OPT_RESET" ]] && { command rm -f "$FAST_WORK_DIR"/{current_theme.zsh,secondary_theme.zsh}; [[ -z "$OPT_QUIET" ]] && print "Reset done (no theme is now set, restart is required)"; return 0; } | |||
[[ -n "$OPT_OV_RESET" ]] && { command rm -f "$FAST_WORK_DIR"/theme_overlay.zsh; [[ -z "$OPT_QUIET" ]] && print "Overlay-reset done, it is inactive (restart is required)"; return 0; } | |||
[[ -n "$OPT_LIST" ]] && { | |||
[[ -z "$OPT_QUIET" ]] && print -r -- "Available themes:" | |||
print -rl -- "$FAST_BASE_DIR"/themes/*.ini(:t:r) | |||
return 0 | |||
} | |||
[[ -n "$OPT_HELP" ]] && { | |||
print -r -- "Usage: fast-theme [-h/--help] [-v/--verbose] [-q/--quiet] [-t/--test] <theme-name|theme-path>" | |||
print -r -- " fast-theme [-r/--reset] [-l/--list] [-s/--show] [-p/--palette] [-w/--workdir]" | |||
print -r -- " fast-theme --copy-shipped-theme {theme-name} [destination-path]" | |||
print -r -- "" | |||
print -r -- "Default action (after providing <theme-name> or <theme-path>) is to switch" | |||
print -r -- "current session and any future sessions to the new theme. Using <theme-path>," | |||
print -r -- "i.e.: a path to an ini file means using custom, own theme. The path can use an" | |||
print -r -- "\"XDG:\" shorthand (e.g.: \"XDG:mytheme\") that will point to ~/.config/fsh/<theme>.ini" | |||
print -r -- "(or \$XDG_CONFIG_HOME/fsh/<theme>.ini in general if the variable is set in the" | |||
print -r -- "environment). If the INI file pointed in the path is \"*overlay*\", then it is" | |||
print -r -- "not a full theme, but an additional theme-snippet that overwrites only selected" | |||
print -r -- "styles of the main theme." | |||
print -r -- "" | |||
print -r -- "Other path-shorthands:" | |||
print -r -- "LOCAL: = /usr/local/share/fsh/" | |||
print -r -- "HOME: = $HOME/.fsh/" | |||
print -r -- "OPT: = /opt/local/share/fsh/" | |||
print -r -- "" | |||
print -r -- "-r/--reset - unset any theme, use default highlighting (requires restart)" | |||
print -r -- "-R/--ov-reset - unset overlay, use styles only from main-theme (requires restart)" | |||
print -r -- "-l/--list - list names of available themes" | |||
print -r -- "-t/--test - show test block of code after switching theme" | |||
print -r -- "-s/--show - get and display the theme currently being set" | |||
print -r -- "-p/--palette - just print all 256 colors and exit (useful when creating a theme)" | |||
print -r -- "-w/--workdir - cd into \$FAST_WORK_DIR (if not set, then into the plugin directory)" | |||
print -r -- "-v/--verbose - more messages during operation" | |||
print -r -- "-q/--quiet - no default messages" | |||
print -r -- "" | |||
print -r -- "The option --copy-shipped-theme allows easy copying of one of the 6 shipped" | |||
print -r -- "themes into given destination path. Normal use means changing directory to" | |||
print -r -- "e.g.: ~/.config/fsh, and then issuing e.g.: \`fast-theme --copy-shipped-theme" | |||
print -r -- "clean mytheme', to obtain a template for own new theme." | |||
return 0 | |||
} | |||
[[ -z "$1" ]] && { print -u2 "Provide a theme (its name or path to its file) to switch to, aborting (see -h/--help)"; return 1; } | |||
# FAST_HIGHLIGHT_STYLES key onto ini-file key | |||
map=( | |||
default "-" | |||
unknown-token "-" | |||
reserved-word "-" | |||
subcommand "- reserved-word" | |||
alias "- command builtin" | |||
suffix-alias "- alias command builtin" | |||
builtin "-" | |||
function "- builtin command" | |||
command "-" | |||
precommand "- command" | |||
commandseparator "-" | |||
hashed-command "- command" | |||
path "-" | |||
path_pathseparator "pathseparator" | |||
globbing "- back-or-dollar-double-quoted-argument" # fallback: variable in string "text $var text" | |||
globbing-ext "- double-quoted-argument" # fallback: the string "abc..." | |||
history-expansion "-" | |||
single-hyphen-option "- single-quoted-argument" | |||
double-hyphen-option "- double-quoted-argument" | |||
back-quoted-argument "-" | |||
single-quoted-argument "-" | |||
double-quoted-argument "-" | |||
dollar-quoted-argument "-" | |||
back-or-dollar-double-quoted-argument "- back-dollar-quoted-argument" | |||
back-dollar-quoted-argument "- back-or-dollar-double-quoted-argument" | |||
assign "- reserved-word" | |||
redirection "- reserved-word" | |||
comment "-" | |||
variable "-" | |||
mathvar "- forvar variable" | |||
mathnum "- fornum" | |||
matherr "- incorrect-subtle" | |||
assign-array-bracket "-" | |||
for-loop-variable "forvar mathvar variable" | |||
for-loop-number "fornum mathnum" | |||
for-loop-operator "foroper reserved-word" | |||
for-loop-separator "forsep commandseparator" | |||
exec-descriptor "- reserved-word" | |||
here-string-tri "-" | |||
here-string-text "- subtle-bg" | |||
here-string-var "- back-or-dollar-double-quoted-argument" | |||
secondary "-" | |||
recursive-base "- default" | |||
case-input "- variable" | |||
case-parentheses "- reserved-word" | |||
case-condition "- correct-subtle" | |||
correct-subtle "-" | |||
incorrect-subtle "-" | |||
subtle-separator "- commandseparator" | |||
subtle-bg "- correct-subtle" | |||
path-to-dir "- path" | |||
paired-bracket "- subtle-bg correct-subtle" | |||
bracket-level-1 "-" | |||
bracket-level-2 "-" | |||
bracket-level-3 "-" | |||
global-alias "- alias suffix-alias" | |||
single-sq-bracket "-" | |||
double-sq-bracket "-" | |||
double-paren "-" | |||
optarg-string "- double-quoted-argument" | |||
optarg-number "- mathnum" | |||
) | |||
# In which order to generate entries | |||
local -a order | |||
order=( | |||
default unknown-token reserved-word alias suffix-alias builtin function command precommand | |||
commandseparator hashed-command path path_pathseparator globbing globbing-ext history-expansion | |||
single-hyphen-option double-hyphen-option back-quoted-argument single-quoted-argument | |||
double-quoted-argument dollar-quoted-argument back-or-dollar-double-quoted-argument | |||
back-dollar-quoted-argument assign redirection comment variable mathvar | |||
mathnum matherr assign-array-bracket for-loop-variable for-loop-number for-loop-operator | |||
for-loop-separator exec-descriptor here-string-tri here-string-text here-string-var secondary | |||
case-input case-parentheses case-condition correct-subtle incorrect-subtle subtle-separator subtle-bg | |||
path-to-dir paired-bracket bracket-level-1 bracket-level-2 bracket-level-3 | |||
global-alias subcommand single-sq-bracket double-sq-bracket double-paren | |||
optarg-string optarg-number recursive-base | |||
) | |||
[[ -n "$OPT_VERBOSE" ]] && print "Number of styles available for customization: ${#order}" | |||
# Named colors | |||
local -a color | |||
color=( red green blue yellow cyan magenta black white default ) | |||
# | |||
# Execution starts here | |||
# | |||
local -A out | |||
local THEME_NAME THEME_PATH="$1" | |||
if [[ "$1" = */* || "$1" = (XDG|LOCAL|HOME|OPT):* ]]; then | |||
1="${${1/(#s)XDG:/${${XDG_CONFIG_HOME:-$HOME/.config}%/}/fsh/}%.ini}.ini" | |||
1="${${1/(#s)LOCAL://usr/local/share/fsh/}%.ini}.ini" | |||
1="${${1/(#s)HOME:/$HOME/.fsh/}%.ini}.ini" | |||
1="${${1/(#s)OPT://opt/local/share/fsh/}%.ini}.ini" | |||
1=${~1} # allow user to quote ~ | |||
[[ ! -f "$1" ]] && { print -u2 "No such theme \`$1', aborting"; return 1; } | |||
[[ ! -r "$1" ]] && { print -u2 "Theme \`$1' unreadable, aborting"; return 1; } | |||
THEME_NAME="${1:t:r}" | |||
.fast-read-ini-file "$1" out "" | |||
else | |||
[[ ! -f "$FAST_BASE_DIR/themes/$1.ini" ]] && { print -u2 "No such theme \`$1', aborting"; return 1; } | |||
[[ ! -r "$FAST_BASE_DIR/themes/$1.ini" ]] && { print -u2 "Theme \`$1' unreadable, aborting"; return 1; } | |||
THEME_NAME="$1" | |||
.fast-read-ini-file "$FAST_BASE_DIR/themes/$1.ini" out "" | |||
fi | |||
[[ -z "$OPT_SECONDARY" ]] && { [[ "$THEME_NAME" = *"overlay"* ]] && local outfile="theme_overlay.zsh" || local outfile="current_theme.zsh"; } || local outfile="secondary_theme.zsh" | |||
[[ -z "$OPT_XCHG" && -z "$OPT_OV_XCHG" ]] && command rm -f "$FAST_WORK_DIR"/"$outfile" | |||
# Set a zstyle and a parameter to carry theme name | |||
if [[ -z "$OPT_SECONDARY" && -z "$OPT_XCHG" && -z "$OPT_OV_XCHG" ]]; then | |||
[[ "$THEME_NAME" != *"overlay"* ]] && { | |||
print -r -- 'zstyle :plugin:fast-syntax-highlighting theme "'"$THEME_NAME"'"' >>! "$FAST_WORK_DIR"/"$outfile" | |||
print -r -- 'typeset -g FAST_THEME_NAME="'"$THEME_NAME"'"' >>! "$FAST_WORK_DIR"/"$outfile" | |||
zstyle :plugin:fast-syntax-highlighting theme "$THEME_NAME" | |||
typeset -g FAST_THEME_NAME="$THEME_NAME" | |||
} | |||
elif [[ -z "$OPT_XCHG" && -z "$OPT_OV_XCHG" ]]; then | |||
local FAST_THEME_NAME="$THEME_NAME" | |||
fi | |||
# Store from which file the theme or overlay is being loaded | |||
[[ "$THEME_NAME" != *"overlay" && -z "$OPT_OV_XCHG" ]] && FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}-path]="$THEME_PATH" || FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}-ov-path]="$THEME_PATH" | |||
# Generate current_theme.zsh or secondary_theme.zsh, traversing ini-file associative array | |||
local k kk | |||
local inikey inival result result2 first_val isbg | |||
integer ov_counter=0 first | |||
for k in "${order[@]}"; do | |||
first=1 | |||
for kk in ${(s. .)map[$k]} default; do | |||
[[ "$kk" = "-" ]] && kk="$k" | |||
(( first )) && first_val="$kk" | |||
inikey="${out[(i)<*>_${kk}]}" | |||
[[ -n "$inikey" ]] && { | |||
(( !first )) && [[ -z "$OPT_QUIET" ]] && { | |||
[[ $kk = default ]] && { | |||
[[ "$THEME_NAME" != *"overlay"* ]] && print "Missing style: $first_val" | |||
} || print "For style $first_val, went for fallback style $kk" | |||
} | |||
break | |||
} | |||
first=0 | |||
[[ "$THEME_NAME" = *"overlay"* ]] && break | |||
done | |||
# ORIG: Clear orig-style when loading a new theme, not overlay | |||
[[ -z "$OPT_OV_XCHG" ]] && unset "FAST_HIGHLIGHT_STYLES[orig-style-$k]" | |||
# ORIG: Restore orig-style when loading a new overlay | |||
[[ -n "$OPT_OV_XCHG" && -n "${FAST_HIGHLIGHT_STYLES[orig-style-$k]}" ]] && { FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}$k]="${FAST_HIGHLIGHT_STYLES[orig-style-$k]}"; unset "FAST_HIGHLIGHT_STYLES[orig-style-$k]"; } | |||
# Set only the keys provided in theme | |||
[[ -z "$inikey" ]] && { [[ -z "$OPT_QUIET" && "$THEME_NAME" != *"overlay"* ]] && print "Missing style $first_val"; continue; } | |||
inival="${out[$inikey]}" | |||
if [[ "$k" = "secondary" && -z "$OPT_SECONDARY" && -n "$inival" ]]; then | |||
fast-theme -q --secondary "$inival" | |||
fi | |||
result="" | |||
if [[ $k = secondary ]]; then | |||
result="$inival" | |||
else | |||
for kk in ${(s:,:)inival} | |||
do | |||
if [[ $kk = (none|(no-|)(bold|blink|conceal|reverse|standout|underline)) ]]; then | |||
result+="${result:+,}$kk" | |||
else | |||
isbg=0 | |||
if [[ $kk = bg:* ]]; then | |||
isbg=1 | |||
kk=${kk#bg:} | |||
fi | |||
if [[ $kk = (${(~j:|:)color}) || $kk = [0-9]## || $kk = \#[0-9a-fA-F](#c6,6) ]]; then | |||
result+="${result:+,}" | |||
(( isbg )) && result+="bg=" || result+="fg=" | |||
result+="$kk" | |||
else | |||
print "cannot parse style $k: unknown color or style element $kk" | |||
fi | |||
fi | |||
done | |||
fi | |||
if [[ "$THEME_NAME" = *"overlay"* || -n "$OPT_OV_XCHG" ]]; then | |||
(( ++ ov_counter )) | |||
[[ -z "$OPT_XCHG$OPT_OV_XCHG" ]] && print -r -- ': ${FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}'"$k"']::='"$result"'}' >>! "$FAST_WORK_DIR"/"$outfile" | |||
# ORIG: Save original value of the overwritten style | |||
FAST_HIGHLIGHT_STYLES[orig-style-$k]=${FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}$k]} | |||
# Overwrite theme's style | |||
FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}$k]="$result" | |||
else | |||
[[ -z "$OPT_XCHG$OPT_OV_XCHG" ]] && print -r -- ': ${FAST_HIGHLIGHT_STYLES['"${FAST_THEME_NAME}$k"']:='"$result"'}' >>! "$FAST_WORK_DIR"/"$outfile" | |||
FAST_HIGHLIGHT_STYLES[${FAST_THEME_NAME}$k]="$result" | |||
fi | |||
done | |||
# This can overwrite some of *_STYLES fields | |||
# Re-apply overlay on top of the theme we switched to | |||
[[ "$THEME_NAME" != *"overlay"* ]] && [[ -r "$FAST_WORK_DIR"/theme_overlay.zsh ]] && source "$FAST_WORK_DIR"/theme_overlay.zsh | |||
zcompile $FAST_WORK_DIR/$outfile 2>/dev/null | |||
[[ -z "$OPT_QUIET" ]] && { | |||
if [[ "$THEME_NAME" != *"overlay"* ]]; then | |||
print "Switched to theme \`$THEME_NAME' (current session, and future sessions)" || \ | |||
else | |||
print "Processed the overlay ($ov_counter keys found), it is now active (for current session, and future sessions)" | |||
fi | |||
} | |||
[[ -n "$OPT_TEST" ]] && { | |||
print -zr ' | |||
# Subshell, assignments, math-mode | |||
echo $(cat /etc/hosts |& grep -i "hello337") | |||
local param1="text ${+variable[test]} text ${var} text"; typeset param2='"'"'other $variable'"'"' | |||
math=$(( 10 + HISTSIZ + HISTSIZE + $SAVEHIST )) size=$(( 0 )) | |||
# Programming-like usage, bracket matching - through distinct colors; note the backslash quoting | |||
for (( ii = 1; ii <= size; ++ ii )); do | |||
if [[ "${cmds[ii]} string" = "| string" ]] | |||
then | |||
sidx=${buffer[(in:ii:)\$\(?#[^\\\\]\)]} # find opening cmd-subst | |||
(( sidx <= len + 100 )) && { | |||
eidx=${buffer[(b:sidx:ii)[^\\\\]\)]} # find closing cmd-subst | |||
} | |||
fi | |||
done | |||
# Regular command-line usage | |||
repeat 0 { | |||
zsh -i -c "cat /etc/shells* | grep -x --line-buffered -i '"'/bin/zsh'"'" | |||
builtin exit $return_value | |||
fast-theme -tq default | |||
fsh-alias -tq default-X # alias '"'"'fsh-alias=fast-theme'"'"' works just like the previous line | |||
command -v git | grep ".+git" && echo $'"'"'Git is installed'"'"' | |||
git checkout -m --ours /etc/shells && git status-X | |||
gem install asciidoctor | |||
cat <<<$PATH | tr : \\n > /dev/null 2>/usr/local | |||
man -a fopen fopen-X | |||
CFLAGS="-g -Wall -O0" ./configure | |||
} | |||
' | |||
} | |||
return 0 | |||
# vim:ft=zsh:et:sw=4:sts=4 |
@ -1,8 +0,0 @@ | |||
zsh-syntax-highlighting / highlighters | |||
====================================== | |||
Navigate into the individual highlighters' documentation to see | |||
what styles (`$ZSH_HIGHLIGHT_STYLES` keys) each highlighter defines. | |||
Refer to the [documentation on highlighters](../docs/highlighters.md) for further | |||
information. |
@ -1 +0,0 @@ | |||
../../docs/highlighters/brackets.md |
@ -1,107 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2010-2017 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Define default styles. | |||
: ${ZSH_HIGHLIGHT_STYLES[bracket-error]:=fg=red,bold} | |||
: ${ZSH_HIGHLIGHT_STYLES[bracket-level-1]:=fg=blue,bold} | |||
: ${ZSH_HIGHLIGHT_STYLES[bracket-level-2]:=fg=green,bold} | |||
: ${ZSH_HIGHLIGHT_STYLES[bracket-level-3]:=fg=magenta,bold} | |||
: ${ZSH_HIGHLIGHT_STYLES[bracket-level-4]:=fg=yellow,bold} | |||
: ${ZSH_HIGHLIGHT_STYLES[bracket-level-5]:=fg=cyan,bold} | |||
: ${ZSH_HIGHLIGHT_STYLES[cursor-matchingbracket]:=standout} | |||
# Whether the brackets highlighter should be called or not. | |||
_zsh_highlight_highlighter_brackets_predicate() | |||
{ | |||
[[ $WIDGET == zle-line-finish ]] || _zsh_highlight_cursor_moved || _zsh_highlight_buffer_modified | |||
} | |||
# Brackets highlighting function. | |||
_zsh_highlight_highlighter_brackets_paint() | |||
{ | |||
local char style | |||
local -i bracket_color_size=${#ZSH_HIGHLIGHT_STYLES[(I)bracket-level-*]} buflen=${#BUFFER} level=0 matchingpos pos | |||
local -A levelpos lastoflevel matching | |||
# Find all brackets and remember which one is matching | |||
pos=0 | |||
for char in ${(s..)BUFFER} ; do | |||
(( ++pos )) | |||
case $char in | |||
["([{"]) | |||
levelpos[$pos]=$((++level)) | |||
lastoflevel[$level]=$pos | |||
;; | |||
[")]}"]) | |||
if (( level > 0 )); then | |||
matchingpos=$lastoflevel[$level] | |||
levelpos[$pos]=$((level--)) | |||
if _zsh_highlight_brackets_match $matchingpos $pos; then | |||
matching[$matchingpos]=$pos | |||
matching[$pos]=$matchingpos | |||
fi | |||
else | |||
levelpos[$pos]=-1 | |||
fi | |||
;; | |||
esac | |||
done | |||
# Now highlight all found brackets | |||
for pos in ${(k)levelpos}; do | |||
if (( $+matching[$pos] )); then | |||
if (( bracket_color_size )); then | |||
_zsh_highlight_add_highlight $((pos - 1)) $pos bracket-level-$(( (levelpos[$pos] - 1) % bracket_color_size + 1 )) | |||
fi | |||
else | |||
_zsh_highlight_add_highlight $((pos - 1)) $pos bracket-error | |||
fi | |||
done | |||
# If cursor is on a bracket, then highlight corresponding bracket, if any. | |||
if [[ $WIDGET != zle-line-finish ]]; then | |||
pos=$((CURSOR + 1)) | |||
if (( $+levelpos[$pos] )) && (( $+matching[$pos] )); then | |||
local -i otherpos=$matching[$pos] | |||
_zsh_highlight_add_highlight $((otherpos - 1)) $otherpos cursor-matchingbracket | |||
fi | |||
fi | |||
} | |||
# Helper function to differentiate type | |||
_zsh_highlight_brackets_match() | |||
{ | |||
case $BUFFER[$1] in | |||
\() [[ $BUFFER[$2] == \) ]];; | |||
\[) [[ $BUFFER[$2] == \] ]];; | |||
\{) [[ $BUFFER[$2] == \} ]];; | |||
*) false;; | |||
esac | |||
} |
@ -1,36 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
WIDGET=zle-line-finish | |||
BUFFER=': $foo[bar]' | |||
CURSOR=6 # cursor is zero-based | |||
expected_region_highlight=( | |||
) |
@ -1,47 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-3]= | |||
BUFFER=': ((( )))' | |||
CURSOR=2 # cursor is zero-based | |||
expected_region_highlight=( | |||
"3 3 bracket-level-1" | |||
"4 4 bracket-level-2" | |||
"5 5 bracket-level-3" | |||
"7 7 bracket-level-3" | |||
"8 8 bracket-level-2" | |||
"9 9 bracket-level-1" | |||
"9 9 cursor-matchingbracket" | |||
) |
@ -1,33 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=': (x)' | |||
expected_region_highlight=( | |||
) |
@ -1,53 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-3]= | |||
BUFFER=': ({[({[(x)]})]})' | |||
expected_region_highlight=( | |||
"3 3 bracket-level-1" | |||
"4 4 bracket-level-2" | |||
"5 5 bracket-level-3" | |||
"6 6 bracket-level-1" | |||
"7 7 bracket-level-2" | |||
"8 8 bracket-level-3" | |||
"9 9 bracket-level-1" | |||
"11 11 bracket-level-1" | |||
"12 12 bracket-level-3" | |||
"13 13 bracket-level-2" | |||
"14 14 bracket-level-1" | |||
"15 15 bracket-level-3" | |||
"16 16 bracket-level-2" | |||
"17 17 bracket-level-1" | |||
) |
@ -1,42 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
BUFFER='echo ({x}]' | |||
expected_region_highlight=( | |||
"6 6 bracket-error" # ( | |||
"7 7 bracket-level-2" # { | |||
"9 9 bracket-level-2" # } | |||
"10 10 bracket-error" # ) | |||
) |
@ -1,42 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
BUFFER=': {"{x}"}' | |||
expected_region_highlight=( | |||
"3 3 bracket-level-1" | |||
"5 5 bracket-level-2" | |||
"7 7 bracket-level-2" | |||
"9 9 bracket-level-1" | |||
) |
@ -1,45 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-3]= | |||
BUFFER='echo $(echo ${(z)array})' | |||
expected_region_highlight=( | |||
"7 7 bracket-level-1" # ( | |||
"14 14 bracket-level-2" # { | |||
"15 15 bracket-level-3" # ( | |||
"17 17 bracket-level-3" # ) | |||
"23 23 bracket-level-2" # } | |||
"24 24 bracket-level-1" # ) | |||
) |
@ -1,34 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2017 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=': x)' | |||
expected_region_highlight=( | |||
"4 4 bracket-error" # ) | |||
) |
@ -1,34 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER='echo "foo ( bar"' | |||
expected_region_highlight=( | |||
"11 11 bracket-error" | |||
) |
@ -1,42 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
BUFFER='echo ({x})' | |||
expected_region_highlight=( | |||
"6 6 bracket-level-1" # ( | |||
"7 7 bracket-level-2" # { | |||
"9 9 bracket-level-2" # } | |||
"10 10 bracket-level-1" # ) | |||
) |
@ -1,41 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-2]= | |||
BUFFER='echo ({x}' | |||
expected_region_highlight=( | |||
"6 6 bracket-error" # ( | |||
"7 7 bracket-level-2" # { | |||
"9 9 bracket-level-2" # } | |||
) |
@ -1,40 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
unsorted=1 | |||
ZSH_HIGHLIGHT_STYLES[bracket-level-1]= | |||
BUFFER='echo {x})' | |||
expected_region_highlight=( | |||
"6 6 bracket-level-1" # { | |||
"8 8 bracket-level-1" # } | |||
"9 9 bracket-error" # ) | |||
) |
@ -1 +0,0 @@ | |||
../../docs/highlighters/cursor.md |
@ -1,47 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2010-2011 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Define default styles. | |||
: ${ZSH_HIGHLIGHT_STYLES[cursor]:=standout} | |||
# Whether the cursor highlighter should be called or not. | |||
_zsh_highlight_highlighter_cursor_predicate() | |||
{ | |||
# remove cursor highlighting when the line is finished | |||
[[ $WIDGET == zle-line-finish ]] || _zsh_highlight_cursor_moved | |||
} | |||
# Cursor highlighting function. | |||
_zsh_highlight_highlighter_cursor_paint() | |||
{ | |||
[[ $WIDGET == zle-line-finish ]] && return | |||
_zsh_highlight_add_highlight $CURSOR $(( $CURSOR + 1 )) cursor | |||
} |
@ -1 +0,0 @@ | |||
../../docs/highlighters/line.md |
@ -1,44 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2010-2011 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Define default styles. | |||
: ${ZSH_HIGHLIGHT_STYLES[line]:=} | |||
# Whether the root highlighter should be called or not. | |||
_zsh_highlight_highlighter_line_predicate() | |||
{ | |||
_zsh_highlight_buffer_modified | |||
} | |||
# root highlighting function. | |||
_zsh_highlight_highlighter_line_paint() | |||
{ | |||
_zsh_highlight_add_highlight 0 $#BUFFER line | |||
} |
@ -1 +0,0 @@ | |||
../../docs/highlighters/main.md |
@ -1,35 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=$'/' | |||
expected_region_highlight=( | |||
'1 1 path_prefix' # / | |||
) |
@ -1,36 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
setopt autocd | |||
BUFFER=$'/' | |||
expected_region_highlight=( | |||
'1 1 autodirectory' # / | |||
) |
@ -1,35 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=$'/bi' | |||
expected_region_highlight=( | |||
'1 3 path_prefix' # /bi | |||
) |
@ -1,37 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=$'/bin; /bin' | |||
expected_region_highlight=( | |||
'1 4 unknown-token' # /bin (in middle) | |||
'5 5 commandseparator' # ; | |||
'7 10 path_prefix' # /bin (at end) | |||
) |
@ -1,38 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
setopt autocd | |||
BUFFER=$'/bin; /bin' | |||
expected_region_highlight=( | |||
'1 4 autodirectory' # /bin (in middle) | |||
'5 5 commandseparator' # ; | |||
'7 10 autodirectory' # /bin (at end) | |||
) |
@ -1,35 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=$'/bin/s' | |||
expected_region_highlight=( | |||
'1 6 path_prefix' # /bin/s | |||
) |
@ -1,35 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER=$'/bin/sh' | |||
expected_region_highlight=( | |||
'1 7 command' # /bin/sh | |||
) |
@ -1,38 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Issue #263 (more-pathological case): aliases[x=y]=z works; the ${(z)} splitter considers | |||
# that a single word; but it's not looked up as an alias. Hence, highlight it as an error. | |||
aliases[x=y]='lorem ipsum dolor sit amet' | |||
BUFFER='x=y ls' | |||
expected_region_highlight=( | |||
"1 3 unknown-token" # x=y | |||
"5 6 default" # ls | |||
) |
@ -1,35 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias foo="echo hello world" | |||
BUFFER="foo" | |||
expected_region_highlight+=( | |||
"1 3 alias" # foo | |||
) |
@ -1,41 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2021 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Have to use cat here as it must be a command that exists. | |||
# Otherwise, the test would fail with the first token being recognized | |||
# as an "unknown-token". | |||
alias ]=cat | |||
BUFFER='] /' | |||
expected_region_highlight=( | |||
'1 1 alias' # ] | |||
'3 3 path' # / | |||
) |
@ -1,37 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# Alias must be at least 4 characters to test the regression | |||
# cf. 139ea2b189819c43cc251825981c116959b15cc3 | |||
alias foobar='echo "$(echo foobar)"' | |||
BUFFER='foobar' | |||
expected_region_highlight=( | |||
"1 6 alias" # foobar | |||
) |
@ -1,37 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# see alias-comment2.zsh and comment-followed.zsh | |||
setopt interactivecomments | |||
alias x=$'# foo\npwd' | |||
BUFFER='x' | |||
expected_region_highlight=( | |||
'1 1 alias' # x | |||
) |
@ -1,37 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# see alias-comment1.zsh | |||
setopt NO_interactivecomments | |||
alias x=$'# foo\npwd' | |||
BUFFER='x' | |||
expected_region_highlight=( | |||
'1 1 unknown-token' # x (#) | |||
) |
@ -1,38 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias x='echo && ls; >' | |||
BUFFER='x file echo' | |||
expected_region_highlight=( | |||
'1 1 alias' # x | |||
'3 6 default' # file | |||
'8 11 builtin' # echo | |||
) |
@ -1,38 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias x='' | |||
BUFFER='x echo foo' | |||
expected_region_highlight=( | |||
'1 1 alias' # x | |||
'3 6 builtin' # echo | |||
'8 10 default' # foo | |||
) |
@ -1,36 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias ls='command ls' | |||
BUFFER='ls' | |||
expected_region_highlight=( | |||
"1 2 alias" # ls | |||
) |
@ -1,36 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias ls=tmp tmp='command ls' | |||
BUFFER='ls' | |||
expected_region_highlight=( | |||
"1 2 alias" # ls | |||
) |
@ -1,43 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias p='print -r --' | |||
BUFFER=$'s=$(p foo)' | |||
expected_region_highlight=( | |||
'1 10 assign' # s=$(p foo) | |||
'3 10 default' # $(p foo) | |||
'3 10 command-substitution-unquoted' # $(p foo) | |||
'3 4 command-substitution-delimiter-unquoted' # $( | |||
'5 5 alias' # p | |||
'7 9 default' # foo | |||
'10 10 command-substitution-delimiter-unquoted' # ) | |||
) |
@ -1,44 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
function b() {} # beware of ALIAS_FUNC_DEF | |||
alias a=b b=c c=b | |||
BUFFER='a foo; :' | |||
expected_region_highlight=( | |||
# An alias is ineligible for expansion whilst it's being expanded. | |||
# Therefore, the "b" in the expansion of the alias "c" is not considered | |||
# as an alias. | |||
'1 1 alias' # a | |||
'3 5 default' # foo | |||
'6 6 commandseparator' # ; | |||
'8 8 builtin' # : | |||
) |
@ -1,35 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias ls="ls" | |||
BUFFER="ls" | |||
expected_region_highlight+=( | |||
"1 2 alias" # ls | |||
) |
@ -1,43 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=b b=sudo | |||
sudo(){} | |||
BUFFER='a -u phy1729 echo; :' | |||
expected_region_highlight=( | |||
'1 1 alias' # a | |||
'3 4 single-hyphen-option' # -u | |||
'6 12 default' # phy1729 | |||
'14 17 builtin' # echo | |||
'18 18 commandseparator' # ; | |||
'20 20 builtin' # : | |||
) |
@ -1,40 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=b b=: | |||
BUFFER='a foo; :' | |||
expected_region_highlight=( | |||
'1 1 alias' # a | |||
'3 5 default' # foo | |||
'6 6 commandseparator' # ; | |||
'8 8 builtin' # : | |||
) |
@ -1,38 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias '$foo'='echo alias' | |||
local foo; foo=(echo param) | |||
BUFFER='$foo' | |||
expected_region_highlight=( | |||
'1 4 alias' # $foo | |||
) |
@ -1,42 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# See also param-precommand-option-argument1.zsh | |||
alias sudo_u='sudo -u' | |||
sudo(){} | |||
BUFFER='sudo_u phy1729 echo foo' | |||
expected_region_highlight=( | |||
'1 6 alias' # sudo_u | |||
'8 14 default' # phy1729 | |||
'17 19 command "issue #540"' # echo (not builtin) | |||
'21 23 default' # foo | |||
) |
@ -1,42 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias sudo_b='sudo -b' | |||
alias sudo_b_u='sudo_b -u' | |||
sudo(){} | |||
BUFFER='sudo_b_u phy1729 echo foo' | |||
expected_region_highlight=( | |||
'1 8 alias' # sudo_b_u | |||
'10 16 default' # phy1729 | |||
'18 21 command "issue #540"' # echo (not builtin) | |||
'23 25 default' # foo | |||
) |
@ -1,42 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
# See also param-precommand-option-argument3.zsh | |||
alias sudo_u='sudo -u' | |||
sudo(){} | |||
BUFFER='sudo_u phy1729 ls foo' | |||
expected_region_highlight=( | |||
'1 6 alias' # sudo_u | |||
'8 14 default' # phy1729 | |||
'16 17 command' # ls | |||
'19 21 default' # foo | |||
) |
@ -1,42 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias sudo_b='sudo -b' | |||
alias sudo_b_u='sudo_b -u' | |||
sudo(){} | |||
BUFFER='sudo_b_u phy1729 ls foo' | |||
expected_region_highlight=( | |||
'1 8 alias' # sudo_b_u | |||
'10 16 default' # phy1729 | |||
'18 19 command' # ls | |||
'21 23 default' # foo | |||
) |
@ -1,39 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) YYYY zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=: ls='ls -l' | |||
BUFFER='"a" foo; \ls' | |||
expected_region_highlight=( | |||
'1 3 unknown-token' # "a" | |||
'5 7 default' # foo | |||
'8 8 commandseparator' # ; | |||
'10 12 command' # \ls | |||
) |
@ -1,38 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias x=\> | |||
BUFFER='x foo echo bar' | |||
expected_region_highlight=( | |||
'1 1 alias' # x | |||
'3 5 default' # foo | |||
'7 10 builtin' # echo | |||
'12 14 default' # bar | |||
) |
@ -1,39 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=: b='a | a' | |||
BUFFER='b | b' | |||
expected_region_highlight=( | |||
'1 1 alias' # b | |||
'3 3 commandseparator' # | | |||
'5 5 alias' # b | |||
) |
@ -1,39 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=: b='a && a' | |||
BUFFER='b && b' | |||
expected_region_highlight=( | |||
'1 1 alias' # b | |||
'3 4 commandseparator' # && | |||
'6 6 alias' # b | |||
) |
@ -1,39 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=: b='a; a' | |||
BUFFER='b; b' | |||
expected_region_highlight=( | |||
'1 1 alias' # b | |||
'2 2 commandseparator' # ; | |||
'4 4 alias' # b | |||
) |
@ -1,42 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=: b='a $(a)' | |||
BUFFER='b $(b)' | |||
expected_region_highlight=( | |||
'1 1 alias' # b | |||
'3 6 default' # $(b) | |||
'3 6 command-substitution-unquoted' # $(b) | |||
'3 4 command-substitution-delimiter-unquoted' # $( | |||
'5 5 alias' # b | |||
'6 6 command-substitution-delimiter-unquoted' # ) | |||
) |
@ -1,43 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=: b='a < <(a)' | |||
BUFFER='b < <(b)' | |||
expected_region_highlight=( | |||
'1 1 alias' # b | |||
'3 3 redirection' # < | |||
'5 8 default' # <(b) | |||
'5 8 process-substitution' # <(b) | |||
'5 6 process-substitution-delimiter' # <( | |||
'7 7 alias' # b | |||
'8 8 process-substitution-delimiter' # ) | |||
) |
@ -1,38 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias echo='echo foo' | |||
BUFFER='echo bar' | |||
expected_region_highlight=( | |||
'1 4 alias' # echo | |||
'6 8 default' # bar | |||
) |
@ -1,37 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias cat='cat | cat' | |||
BUFFER='cat' | |||
expected_region_highlight=( | |||
'1 3 alias' # cat | |||
) |
@ -1,36 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2018 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias x=/ | |||
BUFFER=$'x' | |||
expected_region_highlight=( | |||
'1 1 unknown-token' # x (/) | |||
) |
@ -1,37 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2020 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
setopt autocd | |||
alias x=/ | |||
BUFFER=$'x' | |||
expected_region_highlight=( | |||
'1 1 alias' # x | |||
) |
@ -1,37 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a=b b=foo | |||
BUFFER='a ' | |||
expected_region_highlight=( | |||
'1 1 unknown-token' # a | |||
) |
@ -1,37 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2019 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias a='() { ls "$@" ; foo }' | |||
BUFFER='a ' | |||
expected_region_highlight=( | |||
'1 1 unknown-token' # a | |||
) |
@ -1,53 +0,0 @@ | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2015 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
alias alias1="ls" | |||
alias -s alias2="echo" | |||
function alias1() {} # to check that it's highlighted as an alias, not as a function | |||
BUFFER='x.alias2; alias1; alias2' | |||
# Set expected_region_highlight as a function of zsh version. | |||
# | |||
# Highlight of suffix alias requires zsh-5.1.1 or newer; see issue #126, | |||
# and commit 36403 to zsh itself. Therefore, check if the requisite zsh | |||
# functionality is present, and skip verifying suffix-alias highlighting | |||
# if it isn't. | |||
expected_region_highlight=() | |||
if zmodload -e zsh/parameter || [[ "$(type -w x.alias2)" == *suffix* ]]; then | |||
expected_region_highlight+=( | |||
"1 8 suffix-alias" # x.alias2 | |||
) | |||
fi | |||
expected_region_highlight+=( | |||
"9 9 commandseparator" # ; | |||
"11 16 alias" # alias1 | |||
"17 17 commandseparator" # ; | |||
"19 24 unknown-token" # alias2 | |||
) |
@ -1,41 +0,0 @@ | |||
#!/usr/bin/env zsh | |||
# ------------------------------------------------------------------------------------------------- | |||
# Copyright (c) 2016 zsh-syntax-highlighting contributors | |||
# All rights reserved. | |||
# | |||
# Redistribution and use in source and binary forms, with or without modification, are permitted | |||
# provided that the following conditions are met: | |||
# | |||
# * Redistributions of source code must retain the above copyright notice, this list of conditions | |||
# and the following disclaimer. | |||
# * Redistributions in binary form must reproduce the above copyright notice, this list of | |||
# conditions and the following disclaimer in the documentation and/or other materials provided | |||
# with the distribution. | |||
# * Neither the name of the zsh-syntax-highlighting contributors nor the names of its contributors | |||
# may be used to endorse or promote products derived from this software without specific prior | |||
# written permission. | |||
# | |||
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR | |||
# IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND | |||
# FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR | |||
# CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL | |||
# DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, | |||
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER | |||
# IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT | |||
# OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. | |||
# ------------------------------------------------------------------------------------------------- | |||
# -*- mode: zsh; sh-indentation: 2; indent-tabs-mode: nil; sh-basic-offset: 2; -*- | |||
# vim: ft=zsh sw=2 ts=2 et | |||
# ------------------------------------------------------------------------------------------------- | |||
BUFFER='{ ls } always { pwd }' | |||
expected_region_highlight=( | |||
'1 1 reserved-word' # { | |||
'3 4 command' # ls | |||
'6 6 reserved-word' # } | |||
'8 13 reserved-word' # always | |||
'15 15 reserved-word' # { | |||
'17 19 builtin' # pwd | |||
'21 21 reserved-word' # } | |||
) |